Creating and Publishing Python Packages to PyPI
•8 min read
- Languages, frameworks, tools, and trends
- Skills, interviews, and jobs
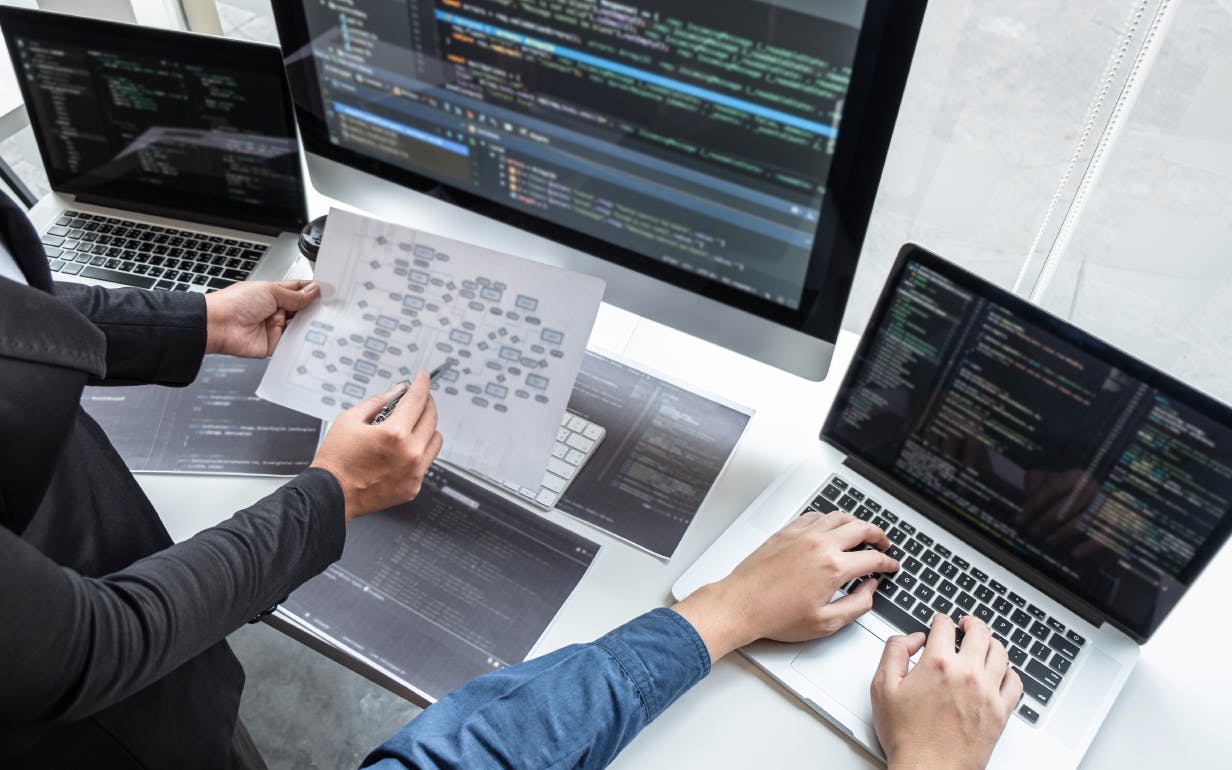
PyPI, short for Python Package Index, is the official third-party package repository for the Python programming language. It is a central repository that hosts and distributes software packages for Python developers to use.
Developers can upload Python packages to PyPI where they can be easily accessed by others. These packages can include libraries, frameworks, utilities, and tools to extend and enhance Python's functionality.
This article will look at the benefits of PyPI, the components of packages, and how to create PyPI packages.
What is PyPI?
PyPI is used to find and install packages for projects. It allows developers to easily manage dependencies and streamline the installation process for their applications. With PyPI, they can save time and effort by reusing existing code rather than writing everything from scratch.
PyPI is maintained by the Python Software Foundation (PSF) and is free to use for developers and end-users. It is an essential component of the Python ecosystem and has contributed to the popularity and growth of the language.
In Python, a package is a way to organize related modules, functions, and classes hierarchically. It provides a structured method to bundle code and can be thought of as a directory containing one or more Python modules. It helps organize code and makes it easier to manage and distribute.
Packages typically include an "init.py" file that is executed when the package is imported. It can define package-level attributes, functions, and classes that can be accessed by other modules in the package. The "init.py" file can also include import statements to import modules and functions from other packages.
Python packages can be installed and managed using package managers like pip which can automatically download and install required dependencies.
With packages, developers can encapsulate related functionality into a single unit, making it easier to reuse and maintain code. For example, the popular NumPy package provides functionality for working with arrays and matrices while the Pandas package provides tools for data analysis and manipulation.
PyPI: A pillar for Python projects
PyPI has a vast collection of libraries, frameworks, and tools that can be installed and integrated into Python projects. Here are some of the benefits:
1. Easy package discovery: Provides a user-friendly website that allows developers to search for and discover new packages that they can use in their projects.
2. Package installation: Makes it easy to install packages using the pip command, which is the de facto standard package installer for Python. This lets developers quickly install and use third-party packages.
3. Package versioning: Maintains a record of all package versions, allowing developers to install and use specific versions of a package.
4. Package dependencies: Allows packages to specify their dependencies on other packages. This ensures that all required packages are installed when a developer installs a particular package.
5. Community support: Maintained by the Python community. It has a vibrant ecosystem of contributors who help maintain and update packages. This means that developers can rely on the community to provide support and updates for the packages they use.
Using PyPI can save developers time and effort by providing access to pre-built functionality that they can incorporate into projects, leading to faster development time and more robust software applications.
Components of a package
The main components of a Python package are:
1. Package directory: Contains the package's modules, including the special "init.py" file.
2. "init.py": A special Python file that is executed when the package is imported. It can contain code to initialize the package as well as definitions for variables, functions, and classes that are accessible to other modules within the package.
3. Module: A Python file that contains code that can be executed or imported by other modules. A package can contain one or more modules.
4. Subpackages: A package can contain one or more sub-packages which are themselves packages that contain their own modules and subpackages.
5. Setup file: Defines metadata about the package, such as its name, version, author, and dependencies. This file is used by package managers like pip to install the package and its dependencies.
6. README file: Provides information about the package, such as its purpose, installation instructions, and usage examples.
7. License file: Specifies the terms under which the package can be used.
Overall, the components of a Python package work together to organize and distribute code in a modular and maintainable way.
How to use PyPI
1. Install pip
To install pip, open a command prompt or terminal and enter the command python -m ensurepip --default-pip. This will install pip if it is not already installed.
2. Search for packages
You can search for packages on PyPI using the pip command pip search "package-name". Replace "package-name" with the name of the package you want to search for.
3. Install packages
To install a package from PyPI, use the pip command pip install "package-name". Replace "package-name" with the name of the package you want to install.
4. Use packages
Once you have installed a package, you can use it in your Python code by importing it. For example, to use the NumPy package, you can add the line ‘import numpy’ to your code.
5. Upgrade packages
To upgrade a package to the latest version available on PyPI, use the pip command pip install --upgrade "package-name". Replace "package-name" with the name of the package you want to upgrade.
6. Uninstall packages
To uninstall a package you no longer need, use the pip command pip uninstall "package-name". Replace "package-name" with the name of the package you want to uninstall.
How to create and upload a package to PyPI
Step 1: Create a project directory
The first step to creating a Python package is to create a new project directory. This will contain all the files and directories necessary for the package.
To create a new project directory, open a terminal or command prompt and run the following command:
mkdir mypackage
cd mypackage
Replace "mypackage" with the name of your package.
Step 2: Create a package directory
The next step is to create a new directory inside the project directory to hold the package code. It should have the same name as your package.
To create a new package directory, run the following command:
mkdir mypackage
Step 3: Create a module file
Inside the package directory, create a new Python file to hold the package code. The file should have the same name as your package with a .py extension.
For example, if your package is named "mypackage", create a file named "mypackage.py".
Step 4: Write the package code
Write your package code in the module file you just created. It can include any Python code you want to have in the package, such as functions, classes, and variables.
Step 5: Create a setup.py file
To publish your Python package to PyPI, you need to create a setup.py file. It contains metadata about your package like its name, version, author, and dependencies.
Create a new file named "setup.py" in the project directory and add the following code:
from setuptools import setup, find_packages
setup(
name='mypackage',
version='0.1.0',
author='Your Name',
author_email='your.email@example.com',
description='A short description of your package',
packages=find_packages(),
classifiers=[
'Programming Language :: Python :: 3',
'License :: OSI Approved :: MIT License',
'Operating System :: OS Independent',
],
python_requires='>=3.6',
)
Step 6: Build the package
To build the package, run the following command in the project directory:
python setup.py sdist
This will create a source distribution of your package in a new directory named "dist".
Step 7: Upload the package to PyPI
To upload your package to PyPI, you first need to create an account on the PyPI website.
Once done, run the following command in the project directory:
twine upload dist/*
This will upload your package to PyPI.
Step 8: Install the package
To install your package from PyPI, run the following command:
pip install mypackage
Creating your own package from scratch
Step 1: Create a project directory
Create a new directory for your project and navigate to it in the terminal:
mkdir mathutils
cd mathutils
Step 2: Create a package directory
Create a new directory inside the project directory for your package:
mkdir mathutils
Step 3: Create module files
Create two new Python files inside the package directory: "operations.py" and "geometry.py". In "operations.py", define a few basic math operations as functions:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
return a / b
In "geometry.py", define a few functions for calculating the area and circumference of a circle:
import math
def circle_area(radius):
return math.pi * radius ** 2
def circle_circumference(radius):
return 2 * math.pi * radius
Step 4: Create a setup.py file
Create a file in the project directory called "setup.py" to define the package metadata:
from setuptools import setup, find_packages
setup(
name="mathutils",
version="0.1.0",
description="A simple Python package for math operations and geometry calculations",
packages=find_packages(),
classifiers=[
"Programming Language :: Python :: 3",
"License :: OSI Approved :: MIT License",
"Operating System :: OS Independent",
],
python_requires=">=3.6",
)
Step 5: Build the package
Build the package using the following command in the terminal:
python setup.py sdist
This creates a source distribution of the package in a new directory called "dist".
Step 6: Install the package
Install the package using pip:
pip install .
This installs the package in your Python environment.
Step 7: Use the package
In your Python code, import the "mathutils" module and call some of the functions:
import mathutils
print(mathutils.operations.add(2, 3))
print(mathutils.geometry.circle_area(5))
This should output "5" and "78.53981633974483", respectively.
That's it! You've created a simple Python package with multiple modules and used it in your code. Note that this is a demo package. In an actual package, you might have many more modules, functions, and dependencies. However, the basic process is the same.
Conclusion
In conclusion, creating and publishing Python packages to PyPI is an essential skill for any Python developer. By sharing your code with the wider community, you can help others solve problems and build upon your work to create even more powerful tools and applications.
In this article, we explored the process of creating and publishing Python packages step by step, from writing the code and testing and building the package to publishing it on PyPI. We also discussed best practices for packaging, such as specifying dependencies and ensuring compatibility with different versions of Python.
By following these practices and investing the time to properly package and document your code, you can make your package more accessible and user-friendly for others. You can also attract other developers to your project who can contribute to your code, report bugs, and help maintain your package in the long term.
In addition to the technical skills required to create and publish a package, there are also important soft skills to consider. Communication, collaboration, and openness to feedback are crucial for successful package development and maintenance and can help ensure that your package remains relevant and useful as time passes.
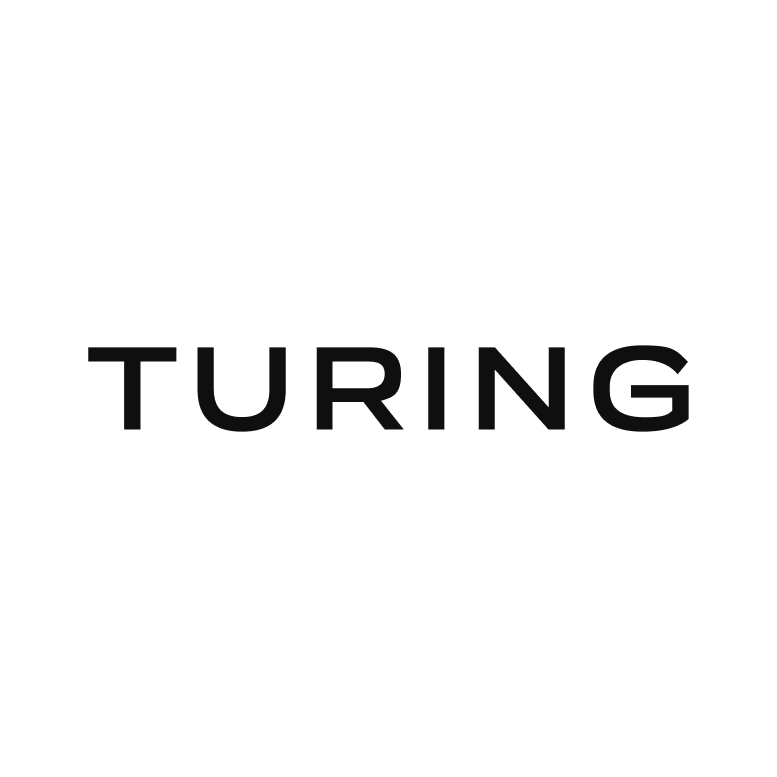
Author
Turing Staff