How to Build a Secure LLM for Application Development Productivity?

Huzefa Chawre
•23 min read
- LLM training and enhancement
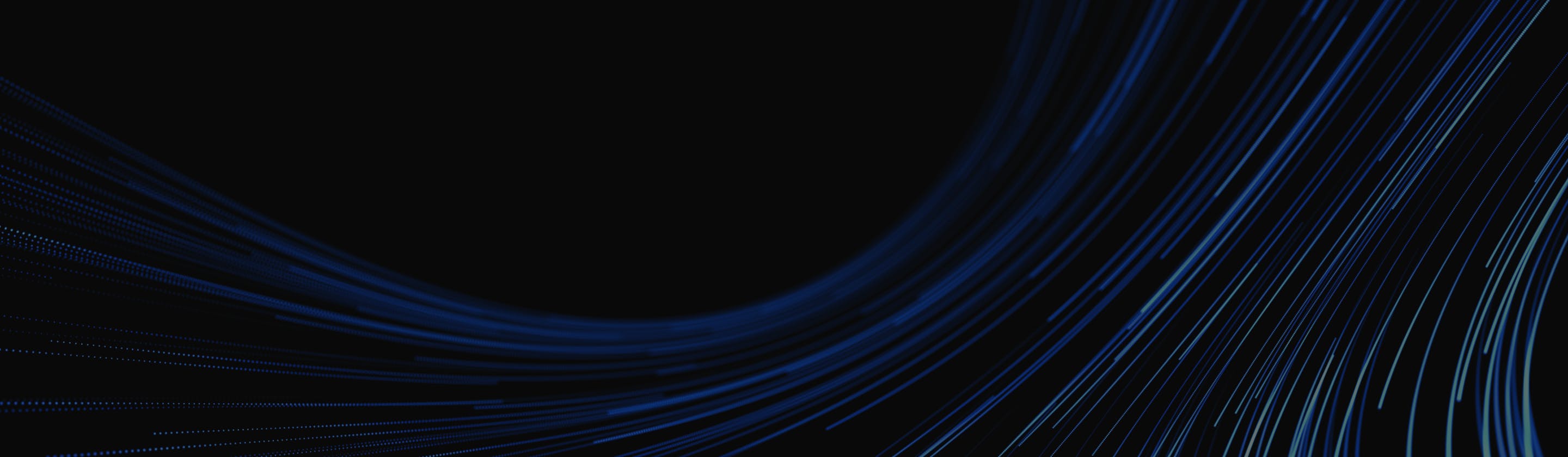
The convergence of generative AI and large language models (LLMs) has created a unique opportunity for enterprises to engineer powerful products and expedite workflows. From crafting compelling content to aiding complex decision-making, LLMs are becoming more powerful and rapidly transforming how businesses operate.
Development modules and coding assistant tools powered by LLMs are shaping the next generation of software products. If you’re in the business of building software applications or digital solutions, then turbocharging your development modules with LLMs development solutions makes perfect business sense.
AI-assisted models offer significantly improved productivity, cost savings, time to market, and scalability. But what exactly is a large language model (LLM), and what are your options for building one?
What is an LLM?
A large language model (LLM) is an artificial intelligence model designed to understand, interpret, and generate human language. These models are trained on large amounts of data, enabling them to learn the structure and semantics of a language.
LLMs use deep learning and natural language processing (NLP) to perform numerous tasks, including text generation, translation, summarization, and sentiment analysis. Prominent examples of LLMs include Google’s Palm-2, OpenAI’s GPT series, and Meta's Llama 2.
So, where do you begin? How do you utilize LLMs for application development?
On a broad scale, you have three options to choose from:
- Use a proprietary model
- Fine-tune an existing model for your specific use case
- Build your own model from scratch
Utilizing an existing LLM is a straightforward approach that allows businesses to harness models like GPT, which are pre-trained on diverse and extensive datasets.
This option benefits companies looking for a quick implementation, as it requires less technical expertise and fewer resources. However, these models may not be tailored to your specific business needs and may not provide the level of customization or data security you need.
On the other hand, fine-tuning an existing model for your specific use cases and requirements offers several advantages. Here’s a quick snapshot of the benefits of an LLM customized for your unique needs:
- Customization: You can train the model on your own datasets, enabling it to understand and generate unique outputs specific to your industry or company.
- Data security: When you customize your model, you have complete control over the data used for training. This is particularly critical for businesses dealing with sensitive information, ensuring you completely own and govern your data.
- Cost-efficiency: Fine-tuning your unique model can be cost-efficient in the long term—you don’t have to pay to use an external service, and you can utilize open-source APIs and tools to build a custom model that can scale based on your evolving needs.
- Innovation: Having your own custom model provides independence from third-party providers. Companies can fully control the development process—including updates, improvements, and feature additions—without relying on external vendors.
Although fine-tuning your custom model requires greater expertise and a longer time frame, it’s a strategic investment that can give businesses a long-term competitive edge in a constantly evolving landscape.
The third option—building your own model from scratch—is only recommended for special scenarios. This approach mandates extensive AI expertise, in-depth research, significant resources, and exorbitant costs that might not be justified (in most cases).
In this article, we broadly cover building a custom LLM from existing pre-trained models. We offer a comprehensive roadmap, including detailed steps, the tech stacks needed, safety integration, and finetuning your models for building an LLM-powered AI app.
Choosing the right framework for building the LLM
Choosing the proper framework or orchestration tool can significantly influence the ease of model development, the efficiency of the model, and the security measures you can implement.
The framework you choose orchestrates the entire LLM development life cycle, including data collection, embedding, storage, model training, fine-tuning, logging, API integration, and validation. You can choose from various options, including Python tools (TensorFlow, PyTorch), LangChain, LlamaIndex, Hugging Face Transformer, and ChatGPT.
- LangChain: LangChain is an open-source framework for developing applications with LLMs. It provides many features that make it easier to use LLMs in applications, such as simple API calling, memory management, chaining, and agents to interact with the LLMs.
- Hugging Face Transformers: Hugging Face Transformers is a state-of-the-art library, that provides thousands of pre-trained models that can be applied to text, speech, vision, tabular data, etc. For text, these models perform tasks such as classification, information extraction, summarization, translation, text generation, and more. It also provides tools for training, fine-tuning, and deploying these models.
- Python tools: If you want an entirely custom model, you can go with Python tools/resources. Python is a popular choice due to its simplicity, flexibility, and the vast number of libraries it offers for machine learning and AI. Libraries and frameworks like TensorFlow and PyTorch provide comprehensive tools for building and training LLMs.
Besides these, you will need several other libraries, such as Pandas for data cleaning and analysis, NumPy for data processing, NLTK for text preprocessing, and SQLite & SQLAlchemy for managing databases.
Data preparation
The first step in building a secure LLM is preparing the data needed to train and fine-tune the model for your specific use-case. This phase lays the foundation for the entire LLM development process. Data preparation involves building robust data pipelines for data collection, loading, and preprocessing.
Data pipelines
A data pipeline is a set of processes that moves data from one system to another, typically involving extraction, transformation, and loading stages. You can use a data pipeline to gather and preprocess the data for training the model. The choice of data pipeline depends on factors like the source and format of your data, the preprocessing steps required, and the scale of your data.
- Data collection: The data collection process for building LLMs involves gathering diverse data. This data serves as the training material for the model, allowing it to learn the syntax, semantics, and common patterns in a language. The quality and diversity of the data collected significantly impact the model's performance, making this a crucial step in the development process. You can use the following code to load the relevant libraries for preparing and working with the data:
# Importing necessary libraries import pandas as pd import numpy as np from sklearn.model_selection import train_test_split from tensorflow.keras.preprocessing.text import Tokenizer from transformers import ( AutoTokenizer, DataCollatorForLanguageModeling, PreTrainedTokenizer, Trainer )
- Data loading: The data loading process for building LLMs involves importing the collected data into your development environment to train the model. This data is typically stored in a file or database, and you must load the data into a format your machine learning library can use.
For instance, if your data is in a CSV file, you might use a library like Pandas to load the data into a DataFrame. If your data is in a database, you might use a library like SQLAlchemy to query the database and load the data.
You can use the following code to load the data and format it for your model training:
# Load data from CSV file data = pd.read_csv('data.csv') # Instantiate tokenizer tokenizer = AutoTokenizer.from_pretrained('gpt4') # Tokenize data inputs = tokenizer(data['text'].tolist(), return_tensors='pt', truncation=True, padding=True) # Instantiate data collator data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=False)
- Data preprocessing and tokenization: Data preprocessing involves cleaning and transforming your raw data into a format the model can easily understand. This could involve removing irrelevant information, inserting missing values, and converting text to lowercase.
Tokenization is breaking down the text into smaller units or tokens. In the context of language models, tokens are often individual words or subwords. Each token is then mapped to a unique numerical identifier the model can understand.
You can use the following code to preprocess and tokenize the data:
# Clean the text data def clean_text(text): text = re.sub(r'\n', ' ', text) # replace newline characters with space text = re.sub(r'\s+', ' ', text) # replace multiple spaces with a single space text = text.strip() # remove leading and trailing spaces return text data['text'] = data['text'].apply(clean_text) # Split the data into training and validation sets train_data, val_data = train_test_split(data, test_size=0.2, random_state=42) # Instantiate tokenizer tokenizer = AutoTokenizer.from_pretrained('gpt4') # Tokenize the text data train_encodings = tokenizer(train_data['text'].tolist(), truncation=True, padding=True) val_encodings = tokenizer(val_data['text'].tolist(), truncation=True, padding=True)
This is a sample code for data preprocessing and tokenization. Your actual code would vary based on your specific needs and data. You can utilize platforms such as DataBricks and Snowflake for comprehensively managing your data requirements.
Defining evaluation metrics
Defining evaluation criteria provides a quantifiable measure to assess the performance and effectiveness of the LLM. These metrics help identify areas of improvement and ensure the model meets the desired objectives. Before selecting a suitable pre-trained model, you need to understand how to evaluate its performance. Different models might perform better or worse depending on the specific evaluation metric.
The choice of evaluation metric also guides the selection of the pre-trained model. For example, if your primary metric is accuracy, you might choose a different model than if your primary metric is recall or precision. Discussing evaluation metrics early on can help set expectations for what constitutes "good" performance for a model in your specific application.
Some prominent evaluation metrics used in assessing the model's performance include:
- BERTScore: BERTScore is a text generation evaluation metric that leverages the BERT language model to measure the similarity between two pieces of text. BERTScore doesn’t rely on n-gram matches and considers the contextual embeddings of words, thus capturing the semantic meaning of the text. It computes the cosine similarity between the contextual embeddings of the generated and referenced text, providing a more nuanced evaluation of text generation models. However, it requires more computational resources due to the complexity of BERT.
- MoverScore: MoverScore is an evaluation metric for text generation tasks. It uses Word Mover's Distance (WMD) and BERT embeddings to measure the semantic similarity between the generated text and the reference text. MoverScore doesn't rely on exact word matches but instead considers the semantic meaning of words and sentences. MoverScore allows for many-to-one matching and offers more comprehensive evaluation, but is highly intensive due to the use of BERT embeddings and WMD.
Metrics like BERTScore and MoverScore provide valuable insights, but they have limitations. They either rely on exact word matches or require significant computational resources. Additionally, they may not fully capture the semantic meaning of the text.
Collecting a set of task-specific evaluations can be advantageous to overcome these limitations. The process involves using prompts, context, and expected outputs as references. This approach allows for a more nuanced understanding of the model's performance, as it considers the specific requirements and subtlety of the task at hand, leading to a more accurate and comprehensive evaluation.
Selecting a pre-trained model
Pre-trained models are machine learning models that have been previously trained on a large corpus of text datasets. Pre-training serves as the foundation for subsequent fine-tuning of an LLM.
The pre-training process, often called language modeling, enables the LLM to learn a language’s syntax, semantics, and common patterns. The pre-training phase is typically unsupervised, meaning the model learns from raw text data without specific task labels. This allows the model to learn the broad representation of a language, capturing a wide range of linguistic features and knowledge.
Components of a pre-trained model
The different components of pre-trained models perform numerous tasks including interpreting and processing data and generating relevant outputs. There are four primary components in pre-trained models:
1. Embedding layer: The embedding layer converts input data, typically words or phrases, into numerical vectors. These vectors capture the semantic meaning of the input data, allowing the model to understand and process the data. The embedding layer’s quality significantly impacts the model’s performance, forming the basis for all subsequent computations. Transformer models such as GPT and BERT generate their own robust embeddings during the pre-training phase, and these embeddings are distinct from stand-alone embedding models like Word2Vec and GloVe.
2. Self-attention mechanism: This component allows the model to weigh the importance of different words in a sentence when generating a representation for a particular word. In other words, it allows the model to "pay attention" to different parts of the input when processing each word. The mechanism computes a score for each word's relevance to the current word and then uses these scores to create a weighted combination of all words' representations.
3. Encoder or decoder blocks: These are the main building blocks of the model. Each block consists of a self-attention layer and a feed-forward neural network. An encoder block takes the input data, often in the form of word embeddings, and transforms it into a higher-level representation that captures the context and relationships between words. On the other hand, a decoder block takes this encoded information and generates the output, such as the predicted next word in language modeling. BERT and RoBERTa use multiple encoder blocks stacked on top of each other, while GPT uses decoder blocks.
4. Output layer: The output layer is the final component of a pre-trained model. It takes the high-level representations produced by the encoder or decoder blocks and transforms them into the final output. The nature of this output depends on the specific task—it could be a probability distribution over possible next words for language modeling or a set of class probabilities for classification tasks. The output layer is typically a dense layer followed by a softmax function for normalization.
The pre-trained models can be fine-tuned on a specific task, saving significant time and computational resources. The choice of a pre-trained model depends on the particular requirements of your application. Factors to consider include the size of the model, its performance on benchmark tasks, the resources required for fine-tuning, and the nature of the data it was originally trained on.
For building LLM-powered applications for development productivity, you can choose from different pre-trained models such as GPT, Codex, BERT, RoBERTa, and T5, among others.
Picking a fine-tuning approach
Once a pre-trained model is selected, the next step in building an LLM is picking a fine-tuning approach. Fine-tuning involves adjusting the pre-trained model to perform a specific task. It's a crucial step that can significantly impact the model’s performance on the desired application. Some prominent fine-tuning approaches are:
- LoRA: LoRA stands for low-rank adaptation. It is a parameter-efficient fine-tuning approach that one can use to fine-tune LLMs on a single GPU. LoRA represents the weight updates with two smaller matrices via low-rank decomposition. You can train these new matrices to adapt to new data while keeping the overall number of changes low.
The main advantage of LoRA is that it is much faster and more memory-efficient than full fine-tuning. This makes it a good choice for fine-tuning LLMs on limited resources. LoRA is also effective at improving the performance of LLMs on various tasks, such as text classification, question answering, and summarization. - QLoRA: QLoRA is a fine-tuning approach that uses a 4-bit quantized model to achieve high accuracy with low memory usage. It offers several innovations like 4-bit NormalFloat quantization, double quantization, and paged optimizers.
The 4-bit NormalFloat quantization is a new method for quantizing models that achieve high accuracy with low memory usage. Double quantization further reduces memory usage by quantizing the gradients and weights. Paged optimizers prevent out-of-memory (OOM) errors by transferring data to CPU RAM when the GPU runs out of memory. - Few-shot learning: Prompt few-shot learning is a fine-tuning technique used to train LLMs where the model is given a few examples or "shots” during inference time to learn a new task. These examples are provided as prompts, which are included in the input when asking the model to make a prediction. The idea behind prompt few-shot learning is to guide the model's predictions by providing context and examples directly in the prompt.
For instance, if you want the model to translate English text to French, you might provide a few examples of English sentences and their French translations in the prompt and then ask the model to translate a new English sentence. Prompt few-shot learning can be a powerful technique for adapting LLMs to new tasks when labeled training data is scarce or unavailable. - Basic hyperparameter tuning: Basic hyperparameter tuning is a simple approach to fine-tuning LLM. It involves manually adjusting the model’s hyperparameters, such as the learning rate, batch size, and the number of epochs until you achieve the desired performance.
The main advantage of basic hyperparameter tuning is its relative ease of execution. However, basic hyperparameter tuning can be time-consuming and inefficient. It can take a lot of trial and error to find the optimal set of hyperparameters, and the model’s performance may still be unsatisfactory.
Ultimately, the choice of the fine-tuning approach depends on your objectives, choice of model, computational resources, and the datasets available to you.
Tools for fine-tuning the model
Multiple tools are available to fine-tune the pre-trained model. Some prominent options include:
- PyTorch: PyTorch is a powerful open-source machine learning framework that provides a flexible platform for fine-tuning pre-trained models. It offers a dynamic computational graph that allows for easy modification and optimization of models. PyTorch supports a wide range of pre-trained models and provides functionalities for automatic differentiation and gradient descent, which are crucial for fine-tuning. Its intuitive interface and extensive documentation make it a popular choice among researchers and developers in deep learning and NLP.
- TRL from Hugging Face: Transformer reinforcement learning (TRL) is a comprehensive library designed by Hugging Face to facilitate the training of transformer language models using reinforcement learning. It uses three steps to train and fine-tune the transformer models:
Supervised fine-tuning (SFT): This step fine-tunes the language model on a supervised dataset. You can use SFT to improve the performance of the language model on a specific task, such as question answering or summarization.
Reward modeling (RM): This step trains a model to predict the reward for a given sequence of tokens. One can use RM to train the language model to generate text that is more likely to be rewarded, such as factually or grammatically correct text.
Proximal policy optimization (PPO): This step trains the language model using the proximal policy optimization (PPO) algorithm. PPO is an iterative algorithm that updates the language model's policy to maximize the expected reward.
Once you have prepared the data, chosen an existing pre-trained model, and fine-tuned that model to your specific needs, you need to build an application, powered by your LLM and tailored to your objectives.
Data retrieval and management
Effective data retrieval and management are critical aspects of optimizing the performance of your LLM-powered application. Data retrieval is how the model accesses and uses external information to generate responses to ensure high levels of contextual accuracy and reduced chances of hallucinations. Effective data management involves organizing, storing, and retrieving data to optimize the model's learning and prediction capabilities.
Let’s explore prominent data retrieval and data management methods used in building a powerful and fully optimized LLM-powered app.
a. Retrieval-augmented generation (RAG)
RAG is an effective technique used in LLM development that combines the benefits of pre-trained transformer models and efficient information retrieval systems. Pre-trained models like BERT and GPT have certain limitations where their responses are solely based on the input and pre-training data, without the ability to access or incorporate external information. This can lead to outputs that may lack factual accuracy or context-specific relevance.
RAG essentially augments the model's knowledge base beyond its training data by pulling in external knowledge not present in its initial training data, enabling it to handle a broader range of queries and produce more informed responses. By leveraging external databases, RAG can significantly enhance the model's ability to generate accurate, informative, and contextually appropriate responses.
Fusion-in-decoder (FiD), retrieval-enhanced transformer (RETRO), and Internet-augmented language models are variants of retrieval-augmented generation that use different techniques to incorporate retrieved information into the generation process.
b. Vector databases
Designed to handle vector data efficiently, a vector database stores and retrieves the vector representations of words or phrases generated by the embedding model. These databases enable rapid and accurate retrieval of semantically related information, facilitating various language-related tasks within applications.
Vector databases can be helpful for tasks like finding the most similar words or phrases to a given input based on their vector representations. One of the key features of vector databases is their ability to perform similarity searches. This is crucial for LLMs, as they often need to find the most similar words or sentences in a high-dimensional space to make predictions or generate text.
Some prominent vector databases you can use for your LLM development include Pinecone, Chroma, Waeviate, and pgvector.
Choosing an app hosting platform
Once you train your models, you’ll need the right app to connect and interact with the user. The choice of platform can significantly impact the user experience, scalability, and overall success of the LLM. A couple of options are:
- Vercel: Vercel is a cloud platform for static sites and serverless functions that fits perfectly with modern frameworks like Next.js. It’s designed to provide developers with a seamless workflow for developing and deploying applications.
Vercel offers several features that can be beneficial for hosting LLM applications. It provides instant static deployment and automatic SSL, which are big pluses for security. It also offers serverless functions, which you can use to run your model inference code without having to manage a server. - Streamlit: Streamlit is a simple open-source app framework designed for machine learning projects. It allows you to turn Python scripts into interactive web apps quickly. You can build a fully interactive app with just a few lines of Python code. Streamlit, with its hot-reloading capability, enables automatic updates and reruns of your app whenever code modifications are made, making it an excellent choice for iterative development.
Streamlit also supports direct deployment from GitHub, GitLab, and Bitbucket, further simplifying the app hosting process. Streamlit's compatibility with major Python libraries, including Pandas, NumPy, and TensorFlow, makes it an ideal platform for hosting LLM-powered AI apps.
Implementing security guardrails for LLMs
LLMs are potent tools that can be used for various applications, but they also pose several security risks. Bad actors can use LLMs to generate harmful, biased, or misleading text. They could also create deepfakes or generate code that can exploit vulnerabilities. Therefore, if you want to build a secure LLM for application development, guardrails are critical for protecting LLMs from such misuse. You can use guardrails to:
- Enforce security policies: Guardrails ensure that LLMs only generate text that complies with specific security policies. For example, you could use a guardrail to ensure that LLMs do not generate hateful or discriminatory text.
- Detect and mitigate risks: You can deploy guardrails to detect and mitigate risks associated with LLMs. For example, a guardrail could detect when an LLM is generating text that is likely to be biased or misleading.
- Protect data and systems: Guardrails can also protect data and systems from potential breaches. For example, a guardrail could prevent an LLM from accessing sensitive data or executing code that could harm a system.
Several guardrail tools are available, each with its strengths and weaknesses. Some popular guardrail tools include:
- GuardRails AI: Guardrails AI, a Python package, uses a language-agnostic, human-readable format called RAIL (Reliable AI Markup Language) to specify the structure, type, and quality guarantees for LLM outputs. Guardrails AI can be used with any LLM that supports the RAIL format. Guardrails AI ensures LLM outputs are in a specific format, such as JSON or CSV. The package also ensures the LLM outputs contain the correct data types, such as numbers or strings. The tool can detect and mitigate risks associated with LLM outputs, such as bias or offensive language.
- NeMo Guardrails: NeMo Guardrails, an open-source toolkit developed by NVIDIA, protects LLMs from various security risks. NeMo Guardrails includes features for enforcing security policies, detecting and mitigating risks, and protecting data and systems. NeMo Guardrails is flexible and can be customized to meet the specific needs of different applications. The toolkit can be leveraged to implement robust guardrails for ensuring relevance, safety, and security. NeMo Guardrails is scalable and easy to use, which makes it an attractive choice for developers.
Ultimately, you should integrate safety and security guardrails that are most compatible with your model and offer the best features for building secure LLM applications.
Managing caching and logging
Managing caching and logging is vital for secure LLM model development as it optimizes performance, monitors usage, and ensures accountability in data processing.
a. Caching
Effective caching can significantly boost the performance of your LLM. You can reduce the time and resources spent on repeated computations by storing and reusing frequently accessed data.
Caching stores frequently accessed data in a “cache”—a high-speed data storage layer. When an application needs this data, it first checks the cache to retrieve it faster. Prominent tools used for caching include:
- Redis: Redis is a popular tool for implementing caching in applications. It offers an in-memory data structure store that applications can utilize as a cache, database, and message broker. Redis is known for its high performance, supporting millions of operations per second, and it is highly versatile, supporting various data structures such as strings, hashes, lists, and sets.
- GPTCache: GPTCache is another tool designed explicitly for caching large language models. It provides a distributed caching layer for transformer models like the GPT series, enabling them to scale across multiple machines without sacrificing performance.
b. Logging
Logging records application actions and state changes into a log file. It is a vital component for debugging and monitoring application activities. By providing a detailed chronological record of events leading up to an error or exception, logs can help developers identify and rectify issues more efficiently.
Regular log monitoring can help proactively address issues before they escalate and impact an application's performance or security. Logs also serve as a valuable resource for auditing and compliance. They provide a verifiable trail of actions and changes, which can be crucial for meeting regulatory requirements, investigating security incidents, or resolving disputes.
Some prominent logging tools you can use for monitoring the performance of your LLM are:
- MLFlow: MLFlow, an open-source platform, provides a centralized repository for logging parameters, metrics, and output artifacts from your machine learning experiments. This allows for easy comparison of different runs and helps identify the most effective models.
- Weights & Biases: Weights & Biases (W&B) is a machine-learning platform that provides various tools for tracking, visualizing, and exploring model performance. Weights and biases can be used to log data from LLM-powered apps, such as the input prompts, the output responses, and the execution metrics. This data can then be used to track the performance of the app over time, identify areas for improvement, and share the results with others.
Overall, logging enables developers to effectively monitor their models, swiftly address issues, and ensure optimal performance throughout an application's lifecycle.
Validating the model
Model validation involves assessing its performance to ensure it functions as expected and makes accurate predictions. Validation identifies any potential issues or biases in the model, allowing developers to make necessary weight adjustments and improvements.
One common approach to model validation is splitting the dataset into a training set and a validation set. The model is trained on the training set and then tested on the validation set. This assesses how well the model can generalize to unseen data. Prominent languages/tools used for model validation include LMQL and Guidance.
- LMQL: LMQL (language model query language) allows developers to query the language model in a structured way, making it easier to test the model's understanding and generate specific responses. This can be particularly useful for evaluating how the model comprehends complex linguistic structures.
LMQL can also be used to fine-tune the model, guiding it to improve its responses over time. This structured approach to querying and testing not only enhances the validation process but also contributes to the overall robustness and reliability of the LLM. - Guidance: Guidance is primarily used to validate and control the performance of large language models. It allows developers to control the output of the LLM, ensuring it generates appropriate and useful responses. It can be instrumental in validating the model's behavior and ensuring it aligns with the intended use case.
Guidance allows developers to set specific parameters or rules that the LLM should adhere to when generating responses. This can range from broad guidelines about the language type to more detailed instructions about what content to include or exclude. By using Guidance, developers can ensure that their LLM not only performs accurately but also behaves in a manner consistent with its intended purpose.
By leveraging tools like LMQL and Guidance, developers can effectively validate their models to ensure they perform optimally and securely in application development.
Guidelines (defensive UX)
Guidelines, or defensive UX, are design strategies that anticipate possible user errors, misunderstandings, and misuse of a language model and provide solutions to prevent or handle these situations. Guidelines gracefully address ambiguous or unclear user inputs, providing helpful error messages or asking for clarification instead of generating incorrect responses.
Guidelines aim to create a user-friendly and safe interaction environment with a language model, and their implementation is crucial for building trust with users. You can implement guidelines by adding disclaimers in the user interface or marketing materials to set the right expectations from the offset.
User feedback
Collecting user feedback is critical to optimizing your LLM-powered app’s performance. This feedback offers valuable insights into how users interact with the app, what they find helpful, and where they encounter difficulties or issues. You can use this feedback to identify areas of improvement, guide future updates, and ensure the app meets its users’ expectations.
While evaluation metrics offer a quantitative measure of the model's performance, user feedback offers qualitative insights these metrics might miss. User feedback can also help identify and address potential ethical issues, such as biases in the model's outputs or app misuse. You can use implicit and explicit feedback mechanisms to gather relevant user information.
Conclusion
Building a custom LLM, trained on business-specific datasets and optimized for unique use cases, requires a deep understanding of the transformer architecture and expert management of multiple tech stacks and processes. If you’re looking for support in your LLM development, Turing offers comprehensive application development services, accelerated by its AI expertise and operational excellence, to help clients build custom and secure large language models that adhere to industry standards.
Turing Application Development Services are powered by internal experts and global engineering talent who have provided solutions for hundreds of clients across several industries. Our AI Transformation Services can assist you in building comprehensive LLMs using the best strategy for your business.
Want to accelerate your business with AI?
Talk to one of our solutions architects and get a complimentary GenAI advisory session.
Get Started
Author
Huzefa Chawre
Huzefa is a technical content writer at Turing. He is a computer science graduate and an Oracle-certified associate in Database Administration. Beyond that, he loves sports and is a big football, cricket, and F1 aficionado.