Abstract Class vs Interface in C#: Uses and Differences
•4 min read
- Software comparisons
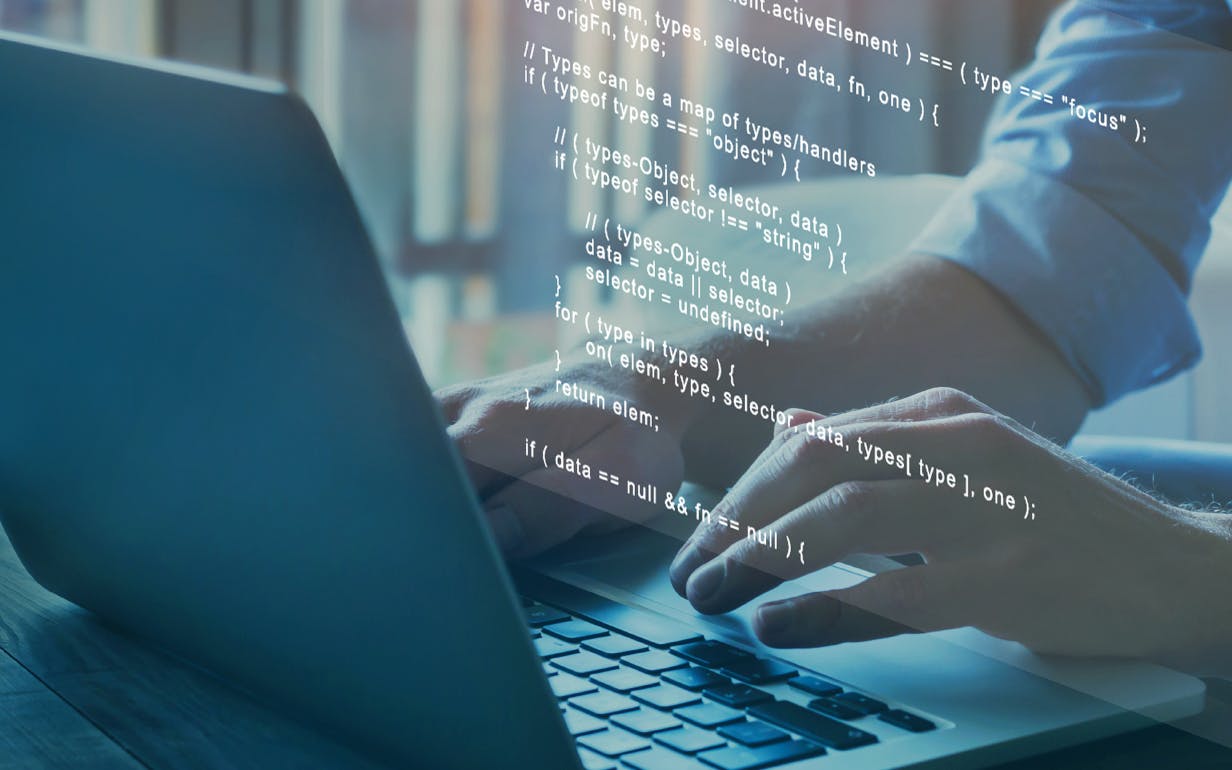
For performing one of the core programming principles, i.e., Abstraction from an object, there are few ways available in object-oriented programming.
Abstraction is the process through which only required data is derived for executing a specific function while disregarding the rest of the accessible data.
Abstract class and interface in C# are used to perform the abstraction; key differences between abstract class and interface in C# are their methods, inheritance, access modifiers, and static members.
In this blog, we will learn when and where to use abstract class and interface in C# to complete tasks at hand.
Both abstract class and interface have their own merits and demerits when performing the same function and producing the same output in a program.
First, let us comprehend the abstract class and interface in C# in detail.
Abstract class in C#
Abstract Class is a way of abstraction in C# that can be created without abstraction methods. However, abstract class in C# can’t be represented/instantiated. The keyword abstract is used for its declaration.
The Abstract class in C# is inherited by subclasses executing or ignoring its methods. So, we can say that the Abstract Class serves as a central class for all subclasses for implementing the functions.
A class library may specify an abstract class that is used as an input to many of its calls, and programmers who use that library may be required to create their class implementation by developing a derived class.
Let's presume you need to create Table, Chair, Bed, and Cupboard objects. They share properties such as color, size, material, and cost. Therefore you establish a Furniture superclass. But what color is the Furniture? What is the serial number on the furniture object?
class Furniture {
// fields and methods
}
// Chair inherits from Furniture
class Chair : Furniture {
// fields and methods of Furniture
// fields and methods of Chair
}
In this scenario, it makes more sense to instantiate subclasses of Furniture rather than an object of type Furniture.
Interface in C#
Interface in C# is another way of abstraction in C#. The interface is used over abstract class in case of multiple inheritances, which we’ll cover later in this blog.
It is declared by using a keyword - interface, possessing only the declaration of the members (methods, events, properties, etc.). The implementation of the interface’s members is given by the class which implements the interface.
- The interface will only specify what a class can do and not how the process will be implemented.
- The interface is used to achieve flexible linkage and complete abstraction.
- The interface is utilized in segment-based application development.
Abstract class vs interface in C#
If both abstract classes and interfaces are used for abstraction in C Sharp, what distinguishes them?
Let's find out!
The scale of the project, decoupling, and scope of future extension are all critical considerations when selecting where to utilize the abstract class and interface in C#.
The interface provides the contract but not the operation; on the other hand, the abstract class provides the execution, the data, and functions that must be adapted.
Abstract class vs. interface: Where you should use abstract class
If large functioning units are not your goal, you can prefer the interface. If you intend to extend the class hierarchy in the future, you should incorporate abstract classes.
Expansion in the class hierarchy while using the abstract class for abstraction in C# is done by only creating a subclass for a parent. However, in the case of an interface, you must construct a new interface for expansion and declare each method in the class.
using System;
// abstract class 'TuringJobs'
public abstract class TuringJobs {
// abstract method 'job()'
public abstract void job();
}
// class 'TuringJobs' inherit in child class 'RemoteJob'
public class RemoteJob : TuringJobs
{
// abstract method 'job()' declared here with 'override' keyword
public override void job()
{
Console.WriteLine("class RemoteJob");
}
}
// class 'TuringJobs' inherits from another child class 'FullTimeJob'
public class FullTimeJob : TuringJobs
{
// same as the previous class
public override void job()
{
Console.WriteLine("class FullTimeJob");
}
}
// Driver Class
public class main_method {
// Main Method
public static void Main()
{
// 't' is the object of class 'TuringJobs'
TuringJobs t;
// instantiate class 'RemoteJob'
t = new RemoteJob();
// call 'job()' of class 'RemoteJob'
t.job();
// instantiate class 'FullTimeJob'
t = new FullTimeJob();
// call 'job()' of class 'FullTimeJob'
t.job();
}
}
Abstract class vs interface: Where you should use interface
Interface in C# requires low coding compared to abstract class for implementation.
If you want to create a plug-and-play architecture in C#, the Interface in C# helps ease the multiple inheritances, which is impossible for an abstract class.
If large functioning units are not your goal, you can prefer to use the interface.
Abstract class vs interface: Major drawbacks
- There can be no static or contained abstract classes.
- Abstract members can only be defined inside the context of an abstract class.
- A concrete class can inherit only one abstract class.
- The template method approach cannot be implemented without an abstract class.
- The interface takes longer to execute than the abstract class.
- When an interface is used for abstraction, the entire implementation is required.
- An interface already implemented in a subclass/derived class is difficult to alter.
C# abstract class and interface: Major differences
Declaration
- Abstract class needs to be declared and defined in the program for its implementation.
- Interface only requires it to be declared as it only represents the objective, not functionality.
Inheritance
- There is only one inheritance possible while using an abstract class for abstraction.
- Multiple inheritances are possible to accomplish by using the interface in C#.
Constructor
- An abstract class has Constructors, which are required when an instance of a class is created.
- The interface doesn’t contain any Constructors.
Access
- The interface only has public access modifiers.
- Abstract Class can contain multiple access modifiers like public, private, or protected.
Static members
- An abstract class can contain static members (only whole members).
- There are no static members in Interface.
Concluding abstract class vs interface
The premise of Abstract class and interface may be puzzling at first. Still, as you learn more about Object Oriented programming, everything begins to come into place.
It all comes down to the requirement of the programmer, what implementation the project requires, which functions are desired to be performed, and how many methods need to be implemented, to figure out what to use – abstract class or interface.
As this blog compiled the uses, merits, and differences between abstract class and interface, Turing’s Developer’s Knowledge hub contain much more learning material and insights for numerous programming languages to help you develop your developer's career.

Author
Mohak Sethi
Mohak is a content writer and strategist. He has developed content in tech, OTT, and travel niches. When he is not writing, he’s probably daydreaming about getting on a spacecraft and exploring the universe.