A detailed guide on unit tests and their advantages
•11 min read
- Languages, frameworks, tools, and trends
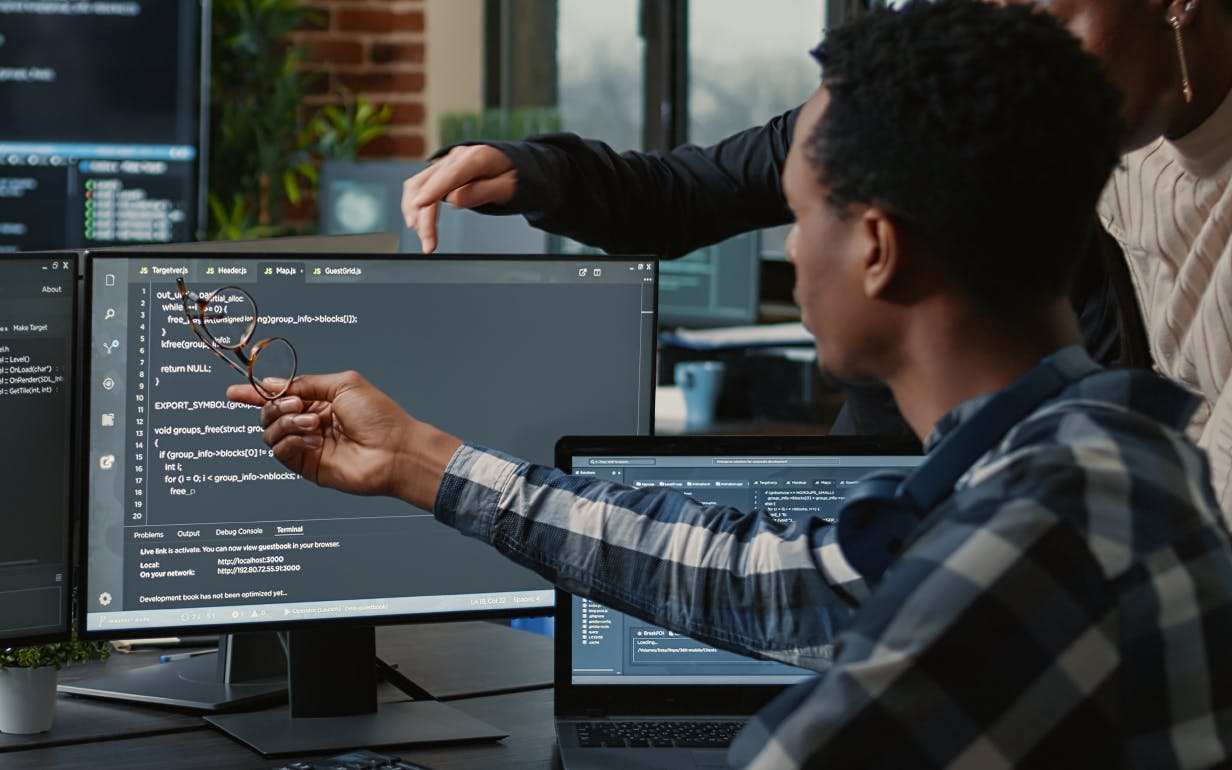
Modern testing approaches have revolutionized the industry, allowing developers to build highly performant and efficient solutions. But even with the widespread use of the technique, developers often face tricky situations while testing code modules. This article has been curated to help you understand the best practices and well-known hurdles related to unit tests.
To avoid tricky situations and build-out services that can efficiently address required processes, understanding the basics of how to do unit testing is essential. Some of the most common reasons holding back the quality of unit testing include poorly-designed/untestable codes and a lack of understanding of the primary goal.
If you find it difficult to build proper unit tests, this guide should feel helpful. Here we'll look into various approaches to help you create deep unit testing mechanisms and understand the best practices related to testing. We will also try to discuss strategies that help make testing codes easier.
But before diving into details, let’s gain a better understanding of unit testing and related processes.
How to do unit testing - explained
Unit testing is an integral part of almost every modern software development process. The minor testable parts of a program, often referred to as a unit, can be tested for performance and scalability-related problems.
Unit tests are conducted mainly by software developers aligned with the project throughout the development cycle in phases. This allows developers to build up services with knowledge about the performance of the existing modules and further requirements.
The primary objective of unit testing is to isolate code modules and test their performance and efficiency. Unit tests can quickly help identify blockers hindering service quality when done correctly and with the right approach.
So, if you’re looking to improve your understanding of unit testing and build tests that address essential areas, the following sections should feel helpful. But before moving ahead, it is vital to have a firm grasp of how unit tests work.
How does unit testing work?
Before creating tests to diagnose individual code modules, it is better to have a thorough understanding of the process. This section should help to improve your knowledge about the same.
A unit test primarily consists of three unique phases -
- Planning,
- Cases, and
- Testing
It starts by initializing a small piece or module of code often referred to as a system under test or SUT. Then the test injects the specific inputs based on SUT requirements and observes the following results.
The results of the unit tests depend on the observed outcome. If observed outcome aligns with the expected behavior, the code module passes the unit tests; all other outputs are considered a failure. As small units tested are intended to initiate or display a single function, any variations in the results should be regarded as unfavorable.
When working on constructing unit tests, you should also keep the importance of mocks in mind. Mocks are essential for conducting a proper unit test. Every test is managed using mock objects, which helps bridge the gaps of missing parts of the final program/software.
For example, a function might need variables or entities that have not been created yet. So you can create mock objects to test the function. But to create and run proper unit tests, you should also be familiar with the different types of procedures and unit testing principles.
Primarily there are three types of unit testing approaches.
- White-Box Testing: The tester is aware of the internal functionalities in white box testing. The internal structure of an item or function to be tested is unknown. It is also referred to as glass box/transparent testing.
- Black-Box Testing: When working with this type of testing procedure, testers don't know the internal functionalities of a system. The internal structure of the function to be tested also remains unknown.
- Gray-Box testing: It's referred to as semi-transparent testing. This works as a combination of a Black and White Box testing approach where the tester is partially aware of the internal functionalities of the method or unit. However, the depth of understanding is not as deep as white box testing. There are also various types of testing (Matrix, Pattern, Regression, etc.) covered under a Gray-box approach.
Now that we have discussed different types of unit tests, we should also look into the advantages of unit testing. It should help you understand how testing small blocks of codes/individual functions can help streamline a software development process.
Benefits of performing unit tests
A streamlined development process
One of the primary benefits of unit testing is working in a streamlined and agile approach. Developers often require additional time to integrate and synchronize new features to the software. This also requires a design upgrade, which can be an expensive and risky process. Using appropriate unit tests can save a significant amount of time as well as resources and speed up the entire process with improved efficiency.
Quality of code
Unit testing is also well known for helping to improve the quality of code. Testing single functions or small blocks of code, developers can quickly identify the effective blockers or minor errors that might be present in the units before going into integration testing. So, unit testing can be a simple yet effective approach to improving code quality during the initial development phase.
Improve the efficiency of troubleshooting
Unit testing is well known for helping developers identify errors and blockers at an early stage in the development cycle. It allows developers to concentrate on addressing the primary blockers before progressing with the planned development schedule.
Resolving major blockers at an early stage offers several benefits and assures no other part of the software is affected. This, in turn, leads to improved efficiency and reduced downtime, making the process less costly than older testing models.
Helps to adapt faster
A variety of problems can be detected early using unit tests. This makes refactoring of the codebase much easier. Developers should verify the accuracy and efficiency of each unit before passing it on to the next phase of development and is integrated it with other modules.
Easy documentation
One of the most significant advantages of unit testing is maintaining proper process documentation. It considers documentation a crucial part of the software development and testing process, which helps developers gain thorough insights during the entire cycle. Using the documentation, new developers can quickly understand the structure and features of the concerned program and detail about each code module.
Help to improve the design
The ability to test individual functions allows developers to rework and innovate the design. This helps them to quickly gain awareness about the existing structure and the necessary changes required to be done. Such insights allow developers to focus more on the details and help visualize and produce better designs.
Cost-efficient approach
Major faults or minor bugs detected at an early phase can significantly help control the process's budget. If identified in later stages of development, resolving errors often cost a lot more than compared to an early fix. Developers need to rework the entire logic to optimize it with the updated approach. Through unit testing, the cost of bug fixes is reduced considerably.
So, these were some of the most significant advantages of integrating unit testing in software development processes. Developers worldwide are constantly trying to innovate and build better solutions using advanced unit test structures.
But despite the growing adoption of unit testing among developers and modern unit test structures, there are certain limitations that you need to keep in mind. It is always better to adopt methods with complete clarity about potential options.
Some of the most significant limitations of unit testing include:
- Unit testing has been found inefficient when trying to detect integration or interfacing issues between two modules
- Identifying complex errors in the system that can range through multiple modules is difficult using unit testing
- Unit testing is not efficient for testing non-functional factors like user-friendliness, scalability, system performance, and more.
- It can rarely offer clarity about functional efficiency and effectiveness of application with its business requirements.
While performing or building unit tests, you should also try to maintain the best practices as a developer. Try to develop a testing mechanism for the individual modules that helps to address all possible outcomes and provide clarity.
So to help you build better and more efficient testing mechanisms, here are some factors that define a well-built unit test.
What makes a good unit test?
Independent and isolated
The best unit tests are often independent and run as isolated tests diagnosing one thing at a time, mainly with a single assertion. Such tests are rarely found to be relying heavily on adverse effects and can be executed in any preferred order of the tester’s choosing. The best unit tests rarely depend on anything other than the small unit of code or SUT. These tests will not access the global state, the file system, or the database.
Extremely fast
The longer the time required to execute a test, the lesser the chances of running. Developers prefer to integrate unit tests that execute instantly because they’re mostly isolated. However, some common problems that hinder the execution speed of unit tests include trying to access a file system, a database, etc.
Well structured
The best unit tests are always well structured and written using Arrange, Act, and Assert patterns. It allows developers to write every unit test in 3 parts and get better results with deeper insights.
But to develop a testing procedure that aligns with what makes a good unit test, you should also be well acquainted with the industry's preferred unit testing tools. So if you’re wondering about tools that would help you build efficient unit tests, check out the following sections for the best options.
Top 5 unit testing tools in 2025
JUnit
JUnit is a widely used open-source tool that developers often prefer to use for performing unit tests. Built using Java, JUnit is used mainly for creating test-driven environments. It offers several benefits including annotation helping developers to find and define test methods. This can help improve developers' efficiency by providing consistency to the development code and significantly reducing the time required to debug a code module.
NUnit
NUnit is probably one of the most widely used tools for unit testing in the present industry. The software is an open-source service and has been ported from JUnit adding support for every .Net language. The tool has been entirely written using C# and designed to take advantage of and utilize different .Net language features.
TestNG
TestING is another open-source unit testing tool compatible with Java and .Net programming languages. It is often termed an advanced unit testing tool and managed using JUnit and NUnit testing frameworks. It also offers other functionalities like additional annotation, parallel execution, group execution, HTML report, and listener, making it a potent tool for unit testing.
Mockito
Mockito is a mocking framework used for unit testing built using Java. It is an open-source tool built to help developers testable applications. Using Mockito, programmers aim to simplify creating tests by mocking external dependencies and using them in the test code. You can use Mockito with other testing frameworks like TestNG and Junit.
PHPUnit
As the name suggests, PHPUnit is a PHP-based testing tool used by developers globally to test codes. It works as an instance of xUnit architectures and has been developed taking inspiration from JUnit framework. PHPUnit can help generate test results in multiple formats with JSON, JUnit XML, TestDox, and Test anything protocol.
So, hope this helps you build up a decent understanding of how unit tests work and what makes a good unit test. But even after decades of being an essential part of software development processes, setting one up can often be tricky. This is often due to the complexity of the codes that you’re looking to test.
As a developer, you should always try to write code that is easy to manage and can also be quickly broken down into modules for testing. Try staying true to the recommended best practices of writing code to produce testable scripts.
If you’re also interested in knowing more about the history of unit tests, the following section should provide some insights.
A brief history of unit testing
The initial years of computers and programming were quite different, where general debugging and unit testing were similar. With codes being too complex to break down into small modules, testing certain sets of code in isolation was extremely difficult.
Post-1997, with the development of JUnit by Kent Beck, developers had the option of using this development environment plugin to test small pieces of code. Over the past few decades, Unit testing has become an essential part of every software development project, with new approaches being developed every year.
The process of code refactoring and unit testing has produced a test-driven development approach using unit tests as a key part of the development process.
Conclusion
Unit testing has become an integral part of software development processes over the years with new techniques being developed from time to time. Integrating unit tests allow developers to build up solutions with a stable code base and clarity about performance.
As a developer, having expertise in writing unit test codes and performing unit tests significantly improves the quality of solutions developed. Not only do developers have the freedom to test individual functions in an isolated environment, but can also make necessary changes or optimizations to improve the quality of codes used.
The process of unit testing has proven to be an excellent approach to maintaining software quality while also adding the ability to address issues at a very early stage. Unit tests can be deployed in every phase of software development to judge the efficiency and correctness of the functions.
So if you were looking to gain deeper insights about unit testing and work it works, and how to write unit test cases, hope this guide has helped you to clear your doubts.
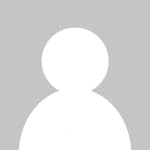
Author
Sayan Sinha
Sayan is a content writer and a contributor to the Turing Blog. Currently working as a content developer and have been a commited sportsman throughout his life.