Understanding JavaScript Modules: Explanations & Examples
•6 min read
- Languages, frameworks, tools, and trends
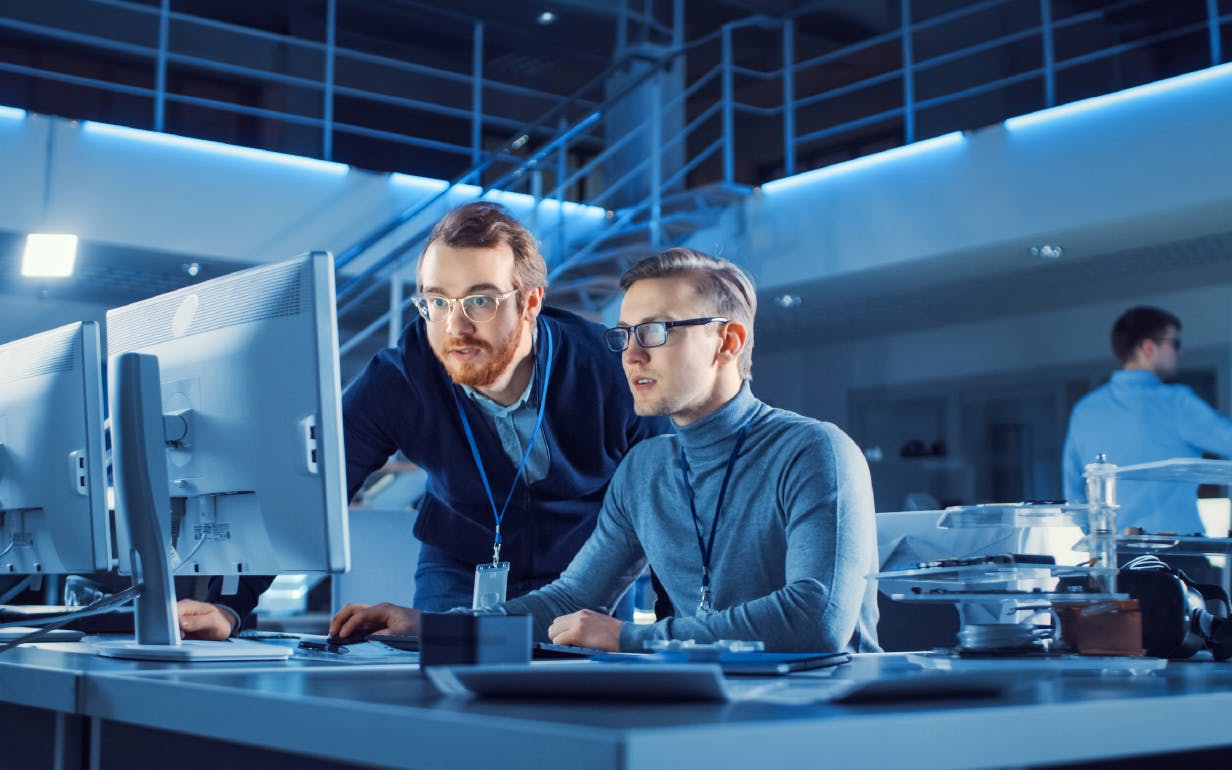
A module is a chunk of code in an external file that performs a specific task or function. It is a separate entity within a program, allowing for modularity and code reusability. By encapsulating related code into modules, developers can organize their programs more efficiently and make them easier to maintain.
Modules also promote code readability and reduce the likelihood of errors, enabling JavaScript developers to focus on specific functionalities without getting overwhelmed by the entire program's complexity.
The benefits of using modules are:
- Reusability: Modules are versatile components that can be reused across multiple programs or applications. This reusability feature not only saves time but also reduces effort.
- Hiding Information: Modules can be used to hide information from other parts of the program, which can improve security and prevent unexpected side effects.
- Code organization: Modules can help you to organize your code in a more logical and maintainable way.
- Encapsulation: Modules can be used to encapsulate functionality, which helps to hide implementation details and make code more robust.
- Collaboration: Modules can make it easier for multiple developers to collaborate on a project since each developer can work on their own module without having to worry about the other modules.
JavaScript modules
In JavaScript, a module is a file that contains code that can be imported into other code files. JavaScript modules are an integral aspect of modern web development. These modules serve as containers for encapsulated pieces of code, allowing developers to organize their projects efficiently. By breaking down the code into smaller, reusable modules, it becomes easier to maintain and update the application over time.
Furthermore, modules enable developers to incorporate external libraries and frameworks seamlessly, enhancing the functionality of their JavaScript code. With the help of modules, developers can create more modular and scalable applications, improving overall code quality. JavaScript modules provide a clear separation of concerns, making it easier for different team members to work on different parts of the codebase simultaneously.
By utilizing the power of JavaScript modules, developers can seamlessly import and export functionalities across various modules, fostering a collaborative environment and facilitating the maintenance of intricate projects. This streamlined approach allows for efficient sharing of code snippets and eliminates the need for redundant coding efforts.
The import and export keywords serve as the bridge that connects different modules, enabling developers to harness the power of modular programming and build scalable solutions. With this modular structure in place, teams can work independently on specific modules while ensuring compatibility and coherence across the entire project. Embracing these keywords empowers developers to write clean, reusable code that promotes efficiency and fosters a sense of unity within development teams.
The export keyword makes variables or functions available to other modules. In contrast, The import keyword is utilized for importing variables or functions into other modules.
Take a look at this example:
//A function that adds two numbers together
function add(num1, num2){
return num1 + num2
}
Assuming the function mentioned above is located within a file named check.js, our objective is to retrieve and utilize it within a separate file.
To do that, the export keyword should be placed in front of the function, and the import keyword should be used to import it into the file where the function is needed.
To utilize a function in another file, the function should be marked with the "export" keyword. This keyword serves as a signal to the program that the function is intended to be accessible from external files. On the file where the function is required, the "import" keyword should be used to import the function. This allows the program to access and utilize the functionality provided by the exported function.
By using the "import" keyword, the program establishes a connection between the two files, enabling the seamless integration of the function into the current file's codebase. Therefore, it is crucial to understand and apply the "export" and "import" keywords correctly when working with functions in separate files.
//adding export in front of the function makes
//it available to the rest of the module
export function isSuperman(name){
if(name==="clark kent"){
return true
}else{
return false
}
}
Now, let's import it into a separate file using the import keyword.
import {isSuperman} from "./check.js"
const result=isSuperman("clark kent")
console.log(result) //true
Typically, This is how JavaScript modules work. However, there is more to the import and export keyword.
Export
As mentioned, the export keyword makes variables or functions available to other modules. We have two types of export: Default and Named Exports.
Named exports
In our previous example, we demonstrated the use of a named export. This particular type of export offers flexibility as it can be utilized in two distinct manners. Firstly, it can be employed individually, allowing for the selective inclusion of specific exports. Alternatively, it can be used collectively, where all the exports are included at the bottom. This provides convenience and ease of access for developers.
Individually:
export const name="clark kent"
export const age=27
export function isSuperman(realName){
if(realName==="clark kent"){
return true;
}else{
return false
}
}
As demonstrated in the code snippet provided, it is worth noting that every variable and function is preceded by the keyword "export." This serves as an indication that each of these elements is individually named.
Collectively at the bottom:
const name="Clark Kent"
const age=27
function isSuperman(realName){
if(realName==="clark kent"){
return true;
}else{
return false
}
}
export {name,age,isSuperman}
In the above code, you will notice that the export does not precede the variable or function. It is written collectively at the bottom.
Default exports
Unlike named export, which can be used multiple times in a file, the default export can only be utilized once. This limitation of the default export sets it apart from the named export. However, the default export can still be employed in two different ways: preceding the code or placing it at the bottom. These two options provide flexibility in how the default export is incorporated into the file.
Preceding:
export default function getRandomNumber(){
return Math.random()
}
At the bottom:
function getRandomNumber(){
return Math.random()
}
export default getRandomNumber
Import
Each export has its own way of being imported. When it comes to importing exports, there are two different approaches depending on whether the export is named or default.
A named export is imported using curly braces, like this:
import { isSuperman } from './otherModule'
isSuperman is the function being imported from another module
On the other hand, a default export is imported without the need for curly braces:
import isSuperman from './otherModule'
Remember to use the appropriate syntax based on the type of export you are working with. To further clarify, it's important to note that a module can have multiple named exports, which can be imported individually or as a group using the asterisk (*) syntax:
import {name, isSuperman} from './otherModule'
Alternatively, you can import all named exports at once using the asterisk (*) syntax:
import * as newlyExport from './otherModule'
console.log(newlyExport) //{name,isSuperman}
The newlyExport serves as the object that has name and isSuperman in it.
Assuming we export a function as default export and two variables as named exports from a file called details.js and import them in a separate file.
export const name="clark kent"
export const age=27
export default function isSuperman(name){
if(name==="clark kent"){
return true;
}else{
return false
}
}
import isSuperman, {name, age} from "./details.js"
const result=isSuperman(name)
console.log(name+" is Superman: "+result)
isSuperman is been imported as a default export, which is why it is not in curly braces while name and age are in curly braces because they are imported as named export.
Tips for using the JavaScript module
Here are some handy tips for effectively using JavaScript modules:
- Use named exports to export specific functions, or variables from your modules. This will make it clear what is being exported from each module and make your code easier to use.
- When exporting code from your modules, it's important to be selective. Avoid the temptation to export everything, as this can make your code harder to maintain. Instead, focus on exporting only what you truly need.
- When developing modules, it is essential to provide clear and concise documentation. This documentation will help other developers understand how to use your modules effectively.
- Default exports should be used to export the main functionality of a module. By using default exports, you can easily export a single value from your module. This can be particularly useful when you want to focus on exporting the main functionality of your module.
- To ensure that your modules are functioning as intended, it is crucial to create and execute unit tests specifically designed for them. This will allow you to verify that each module performs its designated tasks accurately and efficiently.
Conclusion
JavaScript modules are a powerful tool for organizing and structuring code. They allow you to split your code into smaller, self-contained units that can be reused and maintained more easily. Modules also make your code more modular and reusable, which can make it easier for other developers to understand and use.
The import and export keyword is used to share code between modules.
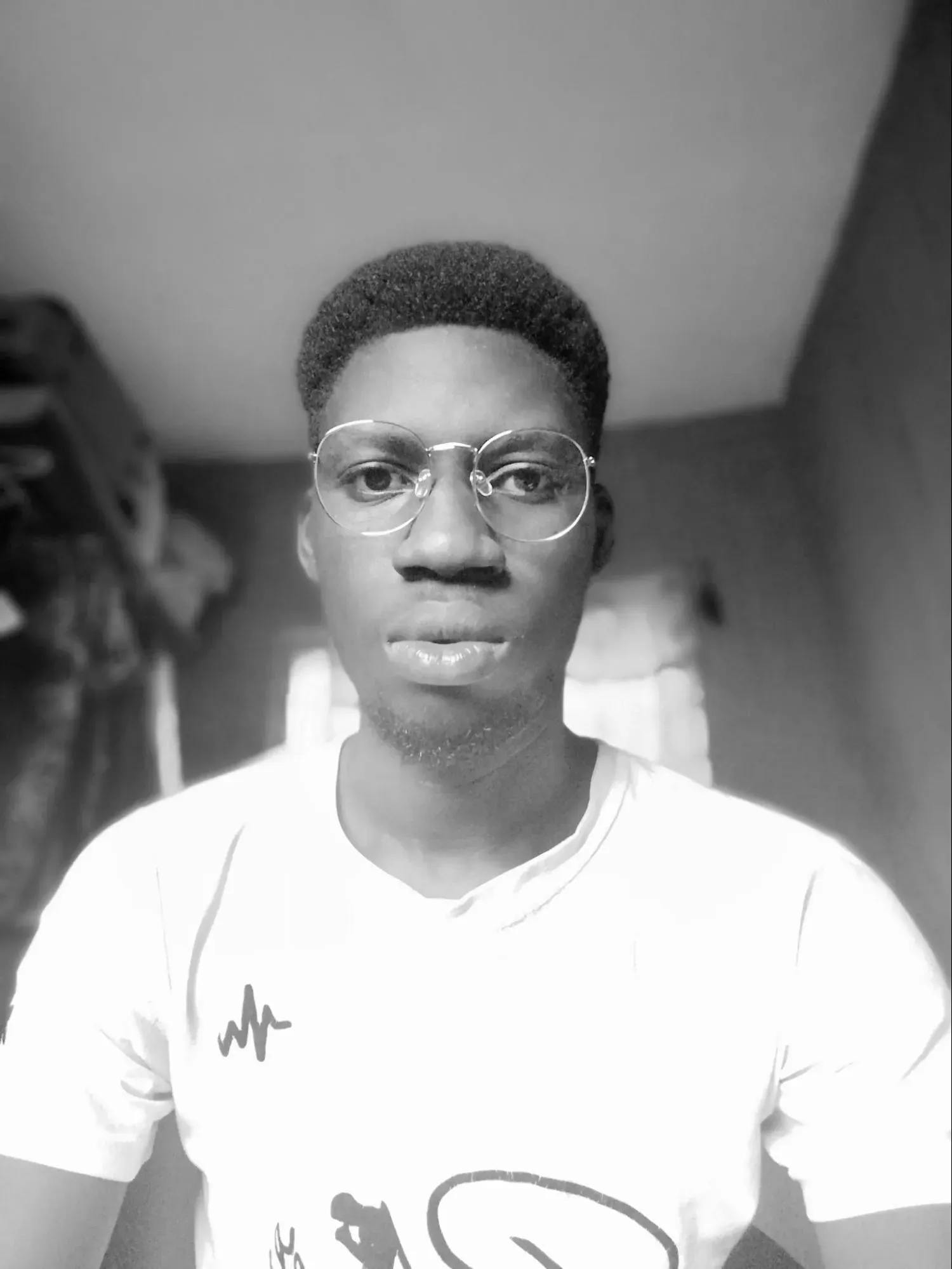
Author
Aremu Olakunle Umaru
Aremu Olakunle is a software engineer who has a passion for technical writing and building web and mobile apps. He is skilled in programming languages such as JavaScript and TypeScript. His stacks are MERN, Next.js, and React-Native. He is constantly expanding his knowledge in the field of software development. With his expertise in both coding and writing, He can effectively communicate complex technical concepts to a wide audience.