Language Server Protocol: Transforming Text Editors Into Code Editors
•6 min read
- Languages, frameworks, tools, and trends
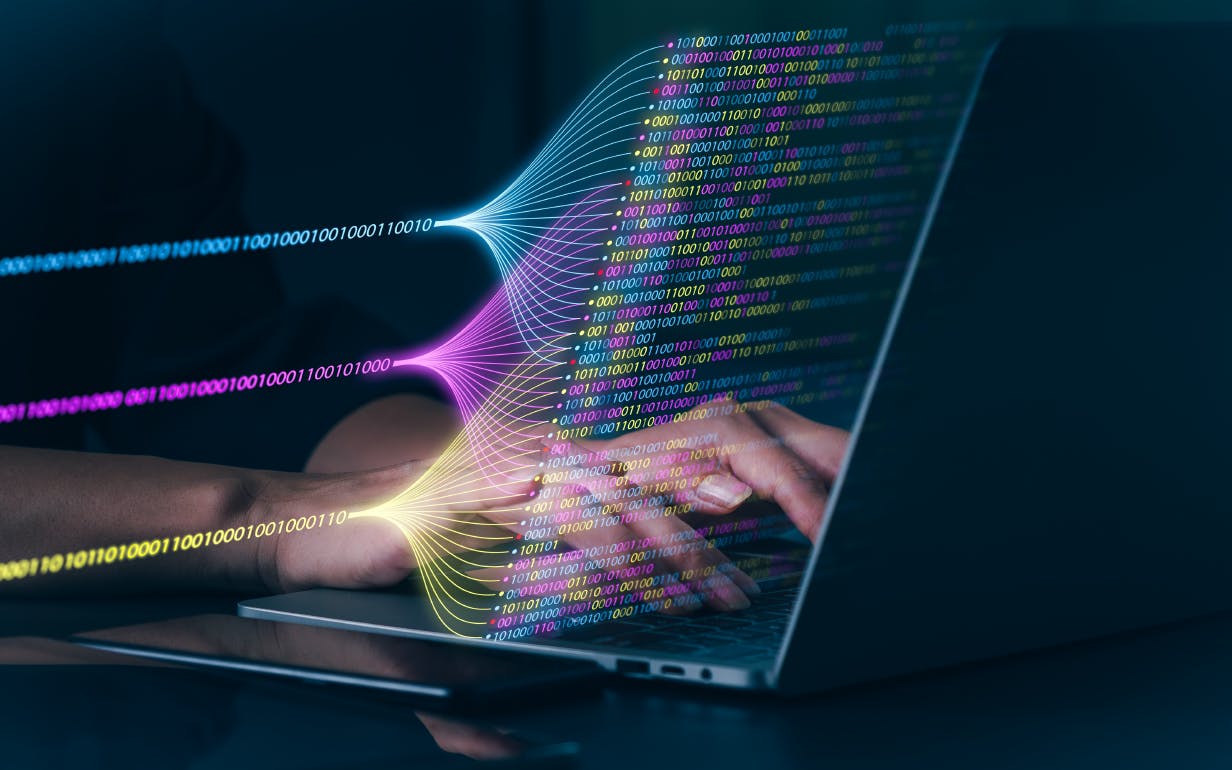
Language server protocol or LSP was created by Microsoft as a communication standard that defines the protocol between the source code editor and the server in a better way. It can be the IDE implemented by the developer or source code editor to a single server that is capable of supporting an array of editors like Eclipse IDE, Visual IDE, and vice versa. These servers are home to all the functions and specifications of a specific programming language.
The main aim behind the design and LSP implementation is to create a fundamental tool that supports languages with dedicated fields for reading and accessing a symbol. However, it cannot operate on a binary code. In this article, we will shed light on the importance of LSP and learn how to implement a language server with a few code examples.
What are language servers?
Language servers are the tools that streamline the communication between language clients and offer insights. They are independent of text editors for programming and provide an additional layer of abstraction. They eliminate the tight coupling between code editors and tools.
With the advent of language servers came language server protocols. Let’s look at them in detail. Language server protocol separates editors and language tools. Although there are several VS Code particularities on these protocols, they are huge steps towards ending the one-size-fits-all approach.
The JSON-RPC protocol defines requests, responses, notifications, and a few basic rules. One of the top features is that it works asynchronously. This provides freedom to the servers and clients to address requests and messages.
To summarize, the JSON-RPC protocol enables a client to request that another program executes a method with parameters and returns an error. LSP performs the following functions:
- Builds the expected data structures.
- Defines different methods.
- Builds rules around the transactions.
The server handles only a single client at a time. There are no limitations on the communication so a language server can easily run on a machine other than the client. LSP offers documentation - if you know what to look for from the heaps of documentation available.
The working of language server protocol
The dedicated process of language server protocol eliminates efforts and repetition to get tasks done in a short amount of time. The example below elaborates how LSP functions.
It all starts when a user opens a document. This is when the editor notifies the server about the action (“textDocument/didOpen”). Here, the document contents are no longer on the file system and are instead kept by the tool in the memory for the entire process.
The next step involves the edits made by the user on the document. The server gets notified (“textDocument/didChange”) about the edits. During this process, the server analyzes the semantic information and notifies possible warnings and errors during the diagnostic process.
Once the edit process is complete, the user requests for a definition for help with the signature or for the completion of the text from the current cursor position. The “Go to definition” on a symbol in the editor executes this request by sending a ‘textDocument/definition' request with two parameters: the text position and the document URI. The user receives the response from the server with the position of the symbols’ definition inside the document and the document URI.
After the completion of the three steps above, the user closes the document which then again sends a notification (“textDocument/didClose”) to the tool. The language server is informed that the current contents are up to date on the file system and are or no longer in the memory.
However, there is a better and more active alternative to the standard way that LSP works:
- The client specifies a particular location in the file and sends this to the server:
‘textDocument/references’. - The server then analyzes the symbol, locates different references, and sends the responses.
- The client displays the results to the user.
This is how LSP communicates with the server and ensures a smooth process.
Types of LSP messages
There are multiple categories defined in LSP. These messages are bifurcated into two main categories:
- Language features
- Admin.
Language features are what we’re most interested in. Diagnostics are one of the key language features. The editor tells if a file is corrupted or will not open. Meanwhile, admin messages contain functions such as opening/altering the files where the servers and clients share common features. They also streamline incremental adoption.
How to create a tool using Vim?
Let’s create a tool and stick to diagnostics instead of creating a new language and distinct features. We will leverage Vim in this example, which is great at editing text. Its plugin ecosystem allows new languages to ramp up quickly.
Starting with Vim
There are two major things to focus on when first starting with VIM:
- Understanding key Vim commands
- Customizing Vim.
Key Vim commands and modes
There are several Vim commands and modes. These features make Vim faster but also pose challenges for beginners.
Understanding the specific mode for specific tasks you want to perform is the key. For the functions listed below, you can enter Normal mode.
Do you want to:
- Save your file?
- Delete multiple lines?
- Navigate to a specific line number?
If you want to write text, you need the Insert mode.
If you want to perform the functions below, you need the Visual mode:
- Highlight multiple lines of single text
- Modify a text block.
After these modes come a few Vim commands.
Note: You will want to brush up on your knowledge by going through a few tutorials for a smoother experience. Enter vimtutor into the terminal command line and go through a few lessons first. After this, you will be ready to run Vim commands.
You can create a new file in Vim with the following command:
vim <filename>.<extension_of_choice>
Customizing Vim
There are two easy ways to customize the Vim setup:
- By enabling the built-in Vim extensions
- By installing external plugins.
Whichever way you go about it, your Vim customization will be the ‘.vimrc’ file, which is the configuration file. You can create the config file in the home directory "~/.vimrc".
Once you are okay with performing the minimum customizations, you will soon get used to working with Vim. Over time, you can add more customizations as you continue to identify the issues. This will improve your efficiency.
Here is the ‘.vimrc’ file:
"""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
" coc extensions
""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
" Extensions listed below will be automatically installed after setup
" of the coc plugin
let g:coc_global_extensions = [
\ 'coc-snippets',
\ 'coc-eslint',
\ 'coc-tsserver',
\ 'coc-html',
\ 'coc-css',
\ 'coc-vimlsp',
\ 'coc-stylelint',
\ 'coc-solargraph',
\ 'coc-json',
\ 'coc-go'
\ ]
"""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
"Vundle
""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
" Installed the package manager Vundle
" Installed from https://github.com/VundleVim/Vundle.vim
" To add plugins, add in the 'Plugin' list then run ':so ~/.vimrc' followed by
" ':PluginInstall'
" Vundle config:
set nocompatible " be iMproved, required
filetype off " required
" set the runtime path to include Vundle and initialize
set rtp+=~/.vim/bundle/Vundle.vim
call vundle#begin()
Plugin 'VundleVim/Vundle.vim'
Plugin 'itchyny/lightline.vim'
Plugin 'NLKNguyen/papercolor-theme'
Plugin 'vim-ruby/vim-ruby'
Plugin 'preservim/nerdtree'
Plugin 'xuyuanp/nerdtree-git-plugin'
Plugin 'jiangmiao/auto-pairs'
Plugin 'tpope/vim-endwise'
Plugin 'plasticboy/vim-markdown'
Plugin 'majutsushi/tagbar'
Plugin 'fatih/vim-go'
" HTML and CSS validation plugin using W3C validator API
Plugin 'arunsahadeo/webval'
" Shows line changes
Plugin 'mhinz/vim-signify'
" Intellisense engine
Plugin 'neoclide/coc.nvim'
" Fuzzy file search
Plugin 'junegunn/fzf'
Plugin 'junegunn/fzf.vim'
Plugin 'airblade/vim-rooter'
Plugin 'honza/vim-snippets'
call vundle#end()
filetype plugin indent on
" Config for vim-go
"let g:go_gpls_enabled =1
" Config for lightline.vim plugin
set laststatus=2
if !has('gui_running')
set t_Co=256
endif
" Config for papercolor theme
set background=dark
set t_Co=256
colorscheme PaperColor
" Enable vim-ruby extensions
syntax on
set nocompatible " We're running Vim, not Vi!
filetype on " Enable filetype detection
filetype indent on " Enable filetype-specific indenting
filetype plugin on " Enable filetype-specific plugins
" Add location of ruby for plugin
"let g:ruby_path = '/home/DAVIEL20/software/miniconda2/envs/ruby/'
" Default enable indent guides from plugin
let g:indent_guides_enable_on_vim_startup = 1
" Enable fly mode for Auto Pairs plugin
let g:AutoPairsFlyMode = 0
let g:AutoPairsShortcutBackInsert = '<M-b>'
" Enable fenced code block languages for vim-markdown plugin
let g:vim_markdown_fenced_languages = ['rb=ruby']
let g:vim_markdown_folding_disabled = 1
" default updatetime 4000ms is not good for async update (signify)
set updatetime=100
" Map the :NERDTree command to ctrl+n
:nnoremap <C-n> :NERDTreeToggle<CR>
" Map tagbar to ctrl+t
nmap <C-t> :TagbarToggle<CR>
" Source config file for coc.nvim
source $HOME/.config/nvim/plug-config/coc.vim
" Automatically run gofmt -w when golang files are saved (not needed with
" vim-go)
"au BufWritePost *.go !gofmt -w %
""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
"General
"""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
" WSL yank support
" Check clipboard support is enabled with `vim --version | grep clipboard` if
" you don't see `+clipboard then install `vim-gtk`.
" let s:clip = '/mnt/c/Windows/System32/clip.exe' " change this path according to your mount point
" if executable(s:clip)
" augroup WSLYank
" autocmd!
" autocmd TextYankPost * if v:event.operator ==# 'y' | call system(s:clip, @0) | endif
" augroup END
" endif
set clipboard=unnamed
"""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
"File settings
""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
"Change default so line numbers are always shown
set number
" Enable syntax highlighting (based on detected filetype)
syntax on
" Change tab to two spaces
set expandtab
set tabstop=2
set shiftwidth=2
set autoindent
set softtabstop=2
" Instead of failing a command because of unsaved changes, instead raise a
" dialogue asking if you wish to save changed files.
set confirm
" Enable highlighting with mouse
set mouse=a
" Enable show white space as dot and tab as arrow
set list
set listchars=tab:→\ ,space:·
" Silence annoying beep sound in WSL
set visualbell
" Allow backspace to delete characters in insert mode
set backspace=indent,eol,start
" Highlight line cursor is on
set cursorline
"""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
" Key maps
""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""""
" map copy from vim clipboard to system clipboard to <F2>
:map <F2> "+y <CR>
" map :set paste to <F3>
:map <F3> :set paste <CR>
" map :set nopaste to <F4>
:map <F4> :set nopaste <CR>
Customizing ‘.vimrc’ files takes time. To avoid remembering multiple customizations at the same time, you can create a dotfiles directory. You can save all your configuration files, such as ‘.bashrc’ and ‘vimrc’, in the dotfiles directory and push them to GitHub.
Note that you will face an issue with Vim here. It will read your "~/.vimrc" file as the default file and not the "~/dotfiles/.vimrc" file.
To make sure that Vim reads your updated "~/dotfiles/.vimrc" each time, you have to create a symbolic link to these two files:
- ~/.vimrc
- ~/dotfiles.vimrc
Establishing a link is easy:
# ln establishes a link; -s flag creates a 'soft' or symbolic link
# ~ is shorthand for your home directory
ln -s ~/dotfiles/.vimrc ~/.vimrc
To check if everything has worked according to your configurations, jump to the home directory and enter ‘ls -lah’. You can use the ‘-lah’ flags and view the files that begin with a dot. You should see something like this:
Image source: Medium
The above code shows that the "~/.vimrc" file is successfully redirected to the "~/dotfiles/.vimrc" file.
The code fragment below shows Vim in action:
Converting text editors into code editors using language server protocols is easy as long as you limit the functionality outside the specific editors. LSP is considered one of the top software development tools owing to its immense support for greater languages as in the past. It has separated the responsibility of the language services from the text editors for programming, which is a clean win.
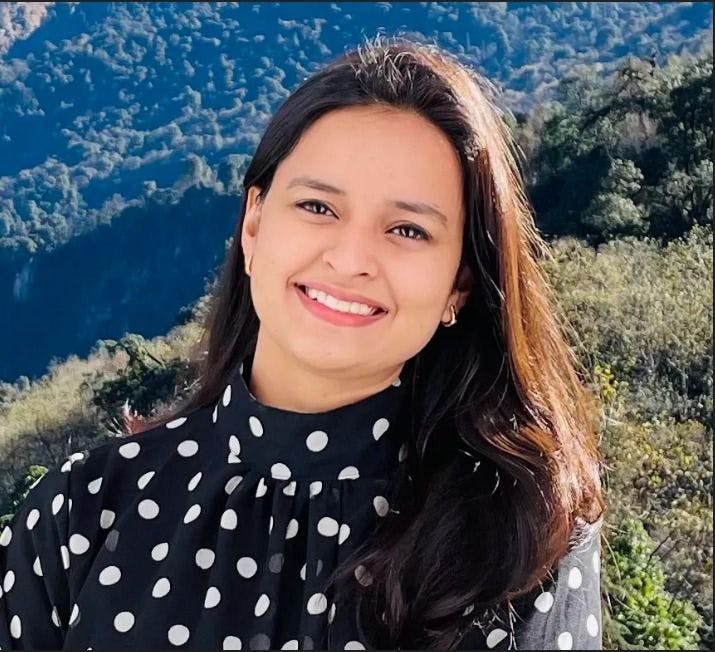
Author
Srishti Chaudhary
Srishti is a competent content writer and marketer with expertise in niches like cloud tech, big data, web development, and digital marketing. She looks forward to grow her tech knowledge and skills.