How to Migrate From JavaScript to TypeScript: A Step-By-Step Guide
•7 min read
- Languages, frameworks, tools, and trends
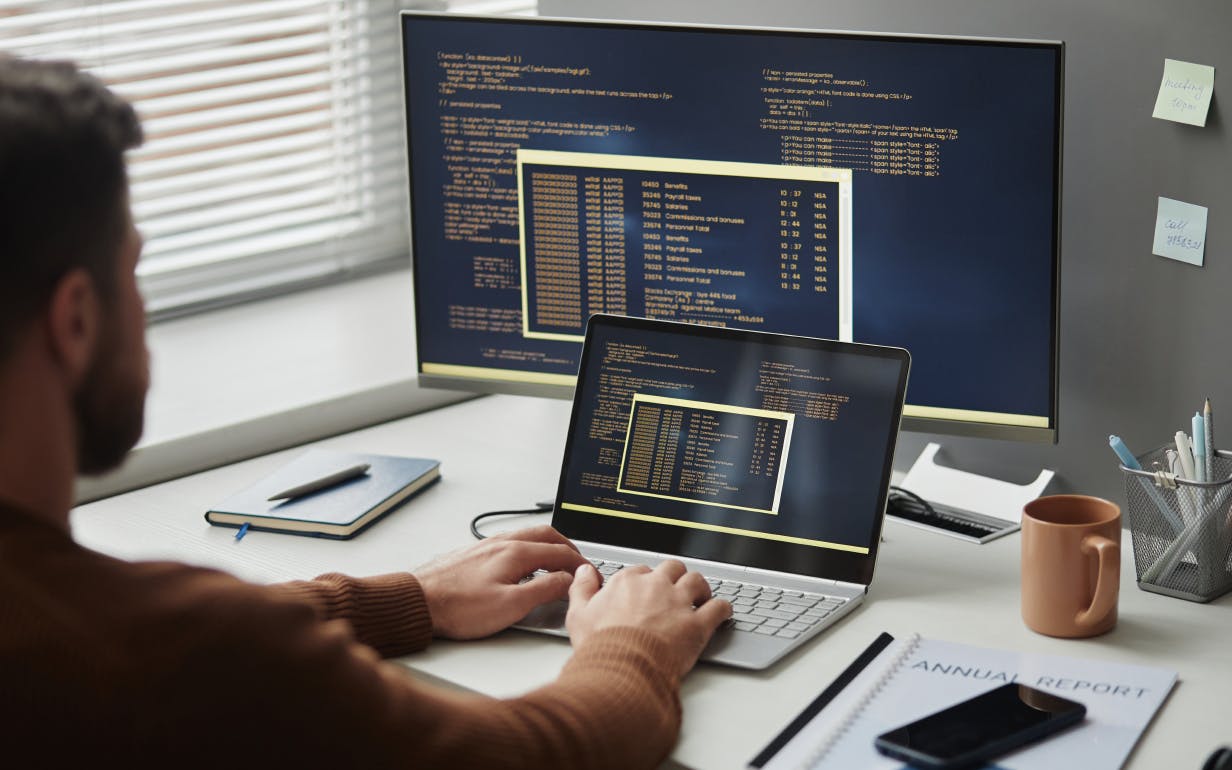
Transitioning from JavaScript to TypeScript might sound like a daunting task. However, it only requires a little preparation and planning. With the right steps and resources, you can make the move with ease.
In this guide, we explain what TypeScript is and show you how to prepare your codebase for the change. We also provide a step-by-step tutorial to help you effortlessly transition from JavaScript to TypeScript.
What is TypeScript and why transition from JavaScript?
TypeScript is a powerful, open-source, typed superset of JavaScript (JS) that allows developers to write code in a typesafe way. It has features like static typing, interfaces, classes, modules, and more. It provides better error-checking and code completion compared to JavaScript, making it easier to debug and maintain code.
TypeScript adds a layer of type annotation to JavaScript, which lets developers define the types of variables and parameters. It provides support for classes, interfaces, modules, and other high-level features that are not available in JavaScript.
It also provides better code completion and syntax highlighting, which makes it easier to identify and fix errors. Additionally, TypeScript has support for typesafe refactoring, which helps to ensure that code changes do not break existing functionality.
Transitioning from JavaScript to TypeScript can provide numerous benefits. It can help developers write robust and maintainable code, improve the speed of development, and make code more scalable. It can also help reduce the amount of time spent debugging and troubleshooting.
Preparing your codebase for transition
Before transitioning from JavaScript to TypeScript, it is important to prepare your codebase. This includes making sure it is up-to-date and that all the necessary tools and libraries are installed. You should also review your existing JavaScript code and decide which parts you want to convert to TypeScript.
It’s vital to ensure that your codebase is properly formatted and that all the variables, functions, and classes are properly named. This will make it easier to identify and convert the relevant parts of the codebase to TypeScript.
Additionally, when reviewing the code, make a note of the TypeScript features, such as classes and modules, that might require you to refactor the code.
Different approaches to transitioning from JavaScript to TypeScript
There are several ways to move from JS to TypeScript.
You can:
- Manually convert your codebase. You will need to go through each file and manually convert the relevant parts. This approach is time-consuming and requires a lot of effort. However, it can provide an opportunity to refactor and clean up the codebase.
- Use a JavaScript to TypeScript converter like TypeScriptify or Babel to automatically convert the codebase. This method is much faster and requires less effort. However, you might need to manually adjust some parts of the code after the conversion process.
Choosing a JavaScript to TypeScript converter
It is important to choose the right converter when transitioning from JS to TypeScript. There are several available, such as TypeScriptify, Babel, and TypeScript Compiler. Each has its strengths and weaknesses, so choose what best fits your needs.
- TypeScriptify is a popular choice as it’s fast, reliable, and easy to use. It supports multiple programming languages and is compatible with most IDEs.
- Babel is also fast, reliable, and supports multiple programming languages. It has built-in support for refactoring, which can help to reduce the amount of time spent debugging and troubleshooting.
- TypeScript Compiler is a powerful converter. It is also fast, reliable, and provides support for refactoring. It also supports typesafe refactoring.
How to transition to TypeScript
When migrating your codebase, an important point to remember is that TypeScript files have a '.ts'extension instead of '.js'. Another is that you can configure your compiler in a way that it accepts '.js' files. This means that you can adopt JavaScript incrementally. This is possible because TypeScript is a superset of JavaScript.
Let’s assume you’ve already started a project in JavaScript.
Step 1: Configure your IDE
Make sure that your IDE is configured to work with Typescript, which will help you with linting and auto-completion. Note that TypeScript has built-in support for Microsoft Visual Studio Code.
Step 2: Add a TypeScript compiler to your project
The easiest way is to install it from npm by running:
npm i typescript
or
yarn add typescript
Step 3: Configure the TypeScript compiler
The fastest way to create the configuration file is by running:
npx tsc –init
When the script finishes, it will create a file called "tsconfig.json" with some default configuration. This file will hold all the compiler configurations. Note that the configuration can become quite complex, but we won’t go too deep into that right now.
There are two properties that you mainly need to focus on:
- "compileOptions"
- "include".
The "compileOptions" object is where you can fine-tune the compiler to your specifications. Common things to configure would be, for instance, "noImplicitAny" or "strictNullChecks". Further, if you add "allowJs: true" to the options, it will accept files with the ".js" extension. This is helpful because it means you can incrementally migrate JavaScript files to TypeScript.
The "include" property holds an array where you can specify the exact filenames or filename patterns that the compiler should pick up. Adding the string ”src/**/*” means that all files in the "src" folder with the extension ".ts", ".tsx", or ".d.ts" will be picked up by the compiler.
Another property to consider is the "exclude" array. Like the "include" property, it tells the compiler what files and folders to ignore. Most commonly, you would add "node_modules" into the excludes array.
You can check the official documentation for all the options.
Step 4: Configure the package.json script
The last step is to add a script into the "package.json" file so you can run the compiler. This will be a little different depending on your project but you basically will want to add a script similar to "tsc:w: tsc -w” which will then compile your project using the "tsconfig.json" file.
Converting a React JavaScript project to TypeScript
If you’re interested in migrating, there’s a possibility that you’re also looking to convert a React project from JavaScript to Typescript. Let’s examine this too.
The steps are basically the same as above:
- Add TypeScript.
- Add tsconfig.json.
- Convert a file to TypeScript.
- Start converting the rest of your files by renaming them from ".jsx" to ".tsx" and ".js" to ".ts".
- Celebrate!
Step 1: Add TypeScript to the project
Let’s assume you have bootstrapped your project using "create-react-app".
Install TypeScript and related types to run the project.
npm i typescript @types/node @types/react @types/react-dom @types/jest
or
yarn add typescript @types/node @types/react @types/react-dom @types/jest
This installs the required packages into your "node_modules".
Step 2: Add the "tsconfig.json" file
As described before, the fastest way is to run the command:
npx tsc -init
This will create the file in the root with a basic configuration.
You will see the output:
Created a new tsconfig.json with:
TS
target: es2016
module: commonjs
strict: true
esModuleInterop: true
skipLibCheck: true
forceConsistentCasingInFileNames: true
You can learn more at https://aka.ms/tsconfig
At this point, only packages have been installed. But in doing so, you’re now ready for the next step.
Step 3: Convert a file to TypeScript
Consider the following component created called "Hero.js". It’s a simple component that has two props, "title" and "subtitle".
const Hero = ({ title, subtitle }) => (
<div>
<h1>{title}</h1>
<p>{subtitle}</p>
</div>
);
export default Hero;
To convert this component, you need to take two actions:
- Change the file extension to ".tsx".
- Add type annotations.
After updating the file extension, the IDE should start showing errors that the props have "any" type. Don’t worry; this is part of the process.
Add the type annotations and update the whole component now called "Hero.tsx".
type Props = {
title: string;
subtitle: string;
};
const Hero: React.FC<Props> = ({ title, subtitle }) => (
<div>
<h1>{title}</h1>
<p>{subtitle}</p>
</div>
);
export default Hero;
Here, you’ve specified that the component is a React functional component with the props title of type string and subtitle of type string (there are different ways to write this but we’ve stuck with this syntax).
If you run the "start"-script, you might see an error that looks like this:
Compiled with problems:X
ERROR in src/Hero.tsx:6:3
TS17004: Cannot use JSX unless the '--jsx' flag is provided.
In this case, go to your "tsconfig.json" file and make the following changes:
{
"compilerOptions": {
…
"jsx": "react-jsx",
…
}
}
The project should compile right away without errors.
Step 4: Update filenames and files
This step is the same process as in step 3 but applies to the rest of your files. If there are many files to migrate, you should consider doing so in smaller batches and incrementally adopting TypeScript.
There might be packages during your migration that do not ship with their own type annotations. If it’s a widely used package, it likely will have a community-maintained "@types/<package-name>" that you can install as a "devDependency".
If there’s no such package, you will need to provide your own annotations. This is often done by creating a "@types" folder in the root of your project and then pointing your TypeScript compiler to look in that folder for types.
A few things to remember:
- If you are using JSX, the extensions need to be ".tsx" and not ".ts".
- While it’s not recommended, TypeScript errors can be suppressed by adding "// @ts-ignore" to the line above the error.
- Keep checking your console for errors and fix them as you work through your project.
- Start small.
Step 5: Celebrate!
If you’ve come this far, pat yourself on the back because you’ve just converted your JavaScript project to TypeScript and will get all the benefits mentioned earlier.
There are a few things to consider, such as increasing the strictness of the compiler. For instance, "noImplicitAny" or "strictNullChecks". This is, of course, up to you. The TypeScript community is growing daily and there are tons of resources on configuring your compiler for best practices.
Conclusion
Some people might try to avoid transitioning from JavaScript to TypeScript due to the perceived complexity. However, once they experience the benefits firsthand, they likely won’t go back. Why not give it a shot yourself and see the amazing advantages that TypeScript provides?
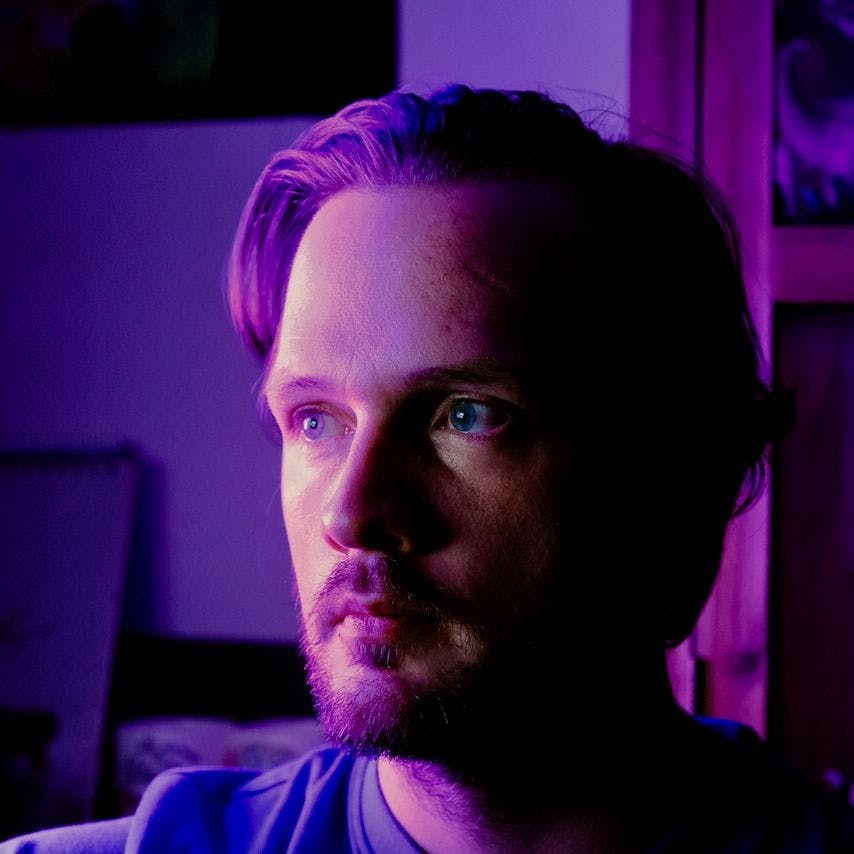
Author
Carl-W. Igelstrom
Carl-W is a Swedish full stack engineer specializing in the JavaScript/TypeScript/React ecosystem, writing web, and mobile applications front to back. He has been shipping production grade code while working 100% remotely to 100s of thousands of users.