Ways to Implement Redirects in Next.js
•3 min read
- Languages, frameworks, tools, and trends
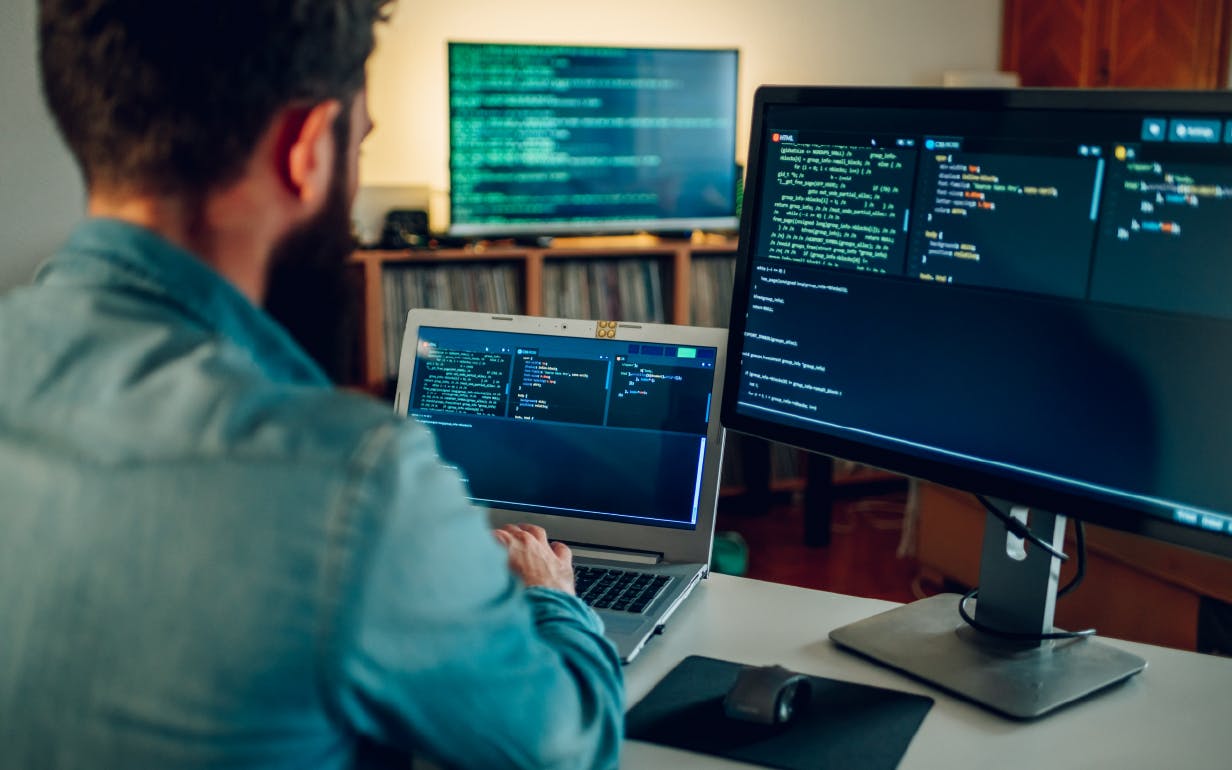
Redirects are essential in web development because they allow us to seamlessly route consumers to alternative pages or URLs. Next.js provides a variety of methods to easily redirect visitors during client-side navigation and implement server-side redirects.
In this detailed article, we will look at numerous approaches for implementing Next.js redirects. We will cover Next.js redirect features, the Next.js router redirect mechanism, Next.js rewrites, and server-side redirects.
Next.js redirect functionality
Next.js includes a 'redirect' method that allows us to programmatically redirect users to another website. This function is frequently used in circumstances like form submissions and authentication flows.
Let's look at a simple example to see how it works:
import { useRouter } from 'next/router';
function MyComponent() {
const router = useRouter();
function handleFormSubmit() {
// Do your stuff..!
// Redirect to the desired page
router.push('/success');
}
return (
<form onSubmit={handleFormSubmit}>
<button type="submit">Submit</button>
</form>
);
}
In the above code snippet, we import the "useRouter" hook from Next.js that grants us access to the router object. Inside the "handleFormSubmit" function, we execute our desired logic and use the "router.push" method to redirect the user to the "/success" page. This approach ensures a smooth client-side navigation experience.
Next.js router redirect
Next.js also makes it simple to do redirects directly from the router. We can smoothly control the navigation flow of an application by setting certain routes and their related redirects.
Consider the following sample scenario:
import { useRouter } from 'next/router';
function AdminPage() {
const router = useRouter();
// Perform some authentication logic
const isAuthenticated = checkIfUserIsAuthenticated();
if (!isAuthenticated) {
// Redirect to the login page
router.replace('/login');
return null; // Render nothing on this page
}
return (
<div>
{/* Admin content */}
</div>
);
}
export default AdminPage;
In the above code, we have an "AdminPage" component that requires authentication. If the user is not authenticated, we utilize the "router.replace" method to redirect them to the login page.
By returning "null" in this case, we prevent the component from rendering any content. This approach provides an efficient way to control access to protected routes.
Next.js rewrites
Custom URL patterns can be defined and mapped to individual pages or external URLs using Next.js rewrites. This functionality is useful for building clean, user-friendly URLs for applications.
Let’s use an example to demonstrate this:
module.exports = {
async rewrites() {
return [
{
source: '/blog/:slug',
destination: '/posts/:slug',
},
{
source: '/google',
destination: 'https://www.google.com',
},
];
},
};
In the above "next.config.js" file, we define two rewrites. The first rewrite maps URLs matching the pattern "/blog/:slug" to the corresponding page in the "/posts" directory. For example, "/blog/hello-world" will be rewritten as "/posts/hello-world". The second rewrite maps the "/google" URL to "https://www.google.com".
With these rewrites, we can effortlessly transform URLs and redirect users to external sites.
Server-side redirects
Next.js also supports server-side redirects, which prove useful when handling redirects based on dynamic data or performing complex logic before redirecting. To implement server-side redirects, we can utilize the "getServerSideProps" function.
Here's an example:
export async function getServerSideProps({ params }) {
const { slug } = params;
// Fetch blog post data based on slug
const post = await fetchPostBySlug(slug);
if (!post) {
// Redirect to a 404 page if the post is not found
return {
redirect: {
destination: '/404',
permanent: false,
},
};
}
return {
props: {
post,
},
};
}
function BlogPost({ post }) {
// Render the blog post content
}
export default BlogPost;
In the above code, the "getServerSideProps" function is responsible for fetching the blog post data based on the provided "slug". If the post is not found, we return a redirect object with the "destination" set to "/404".
By setting the "permanent" property to "false", we indicate a temporary redirect. Finally, the "BlogPost" component receives the fetched "post" as a prop and renders the blog post content.
Conclusion
Building sophisticated online apps requires the ability to implement Next.js redirects. Next.js provides versatile and strong functionality to manage all of these cases, whether we need to redirect users during client-side navigation, protect certain routes with authentication, generate clean URL patterns, or handle redirection server-side.
We can ensure a smooth and flawless user experience by utilizing the 'redirect' function, router redirects, rewrites, and server-side redirects.
Remember that employing redirects correctly can significantly improve the usability and functioning of applications. Begin experimenting with Next.js redirect features to develop interesting web experiences for your users.
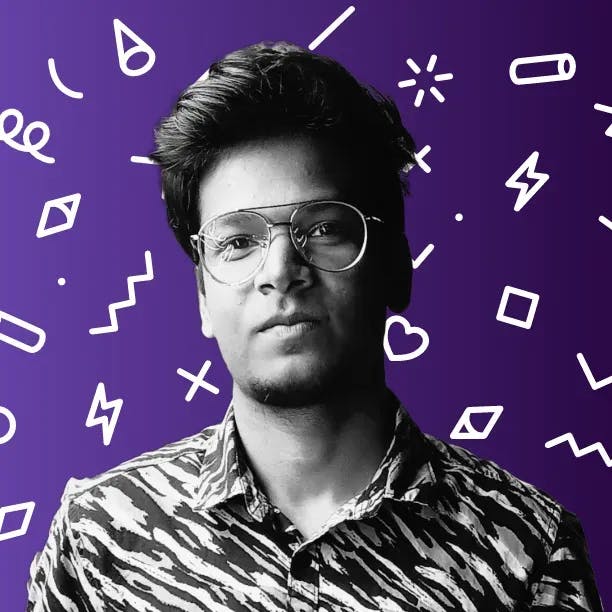
Author
Anas Raza
First-generation solopreneur and full-stack engineer with a passion for building innovative solutions.