Know How to Optimize the Swift Array With ContiguousArray
•5 min read
- Languages, frameworks, tools, and trends
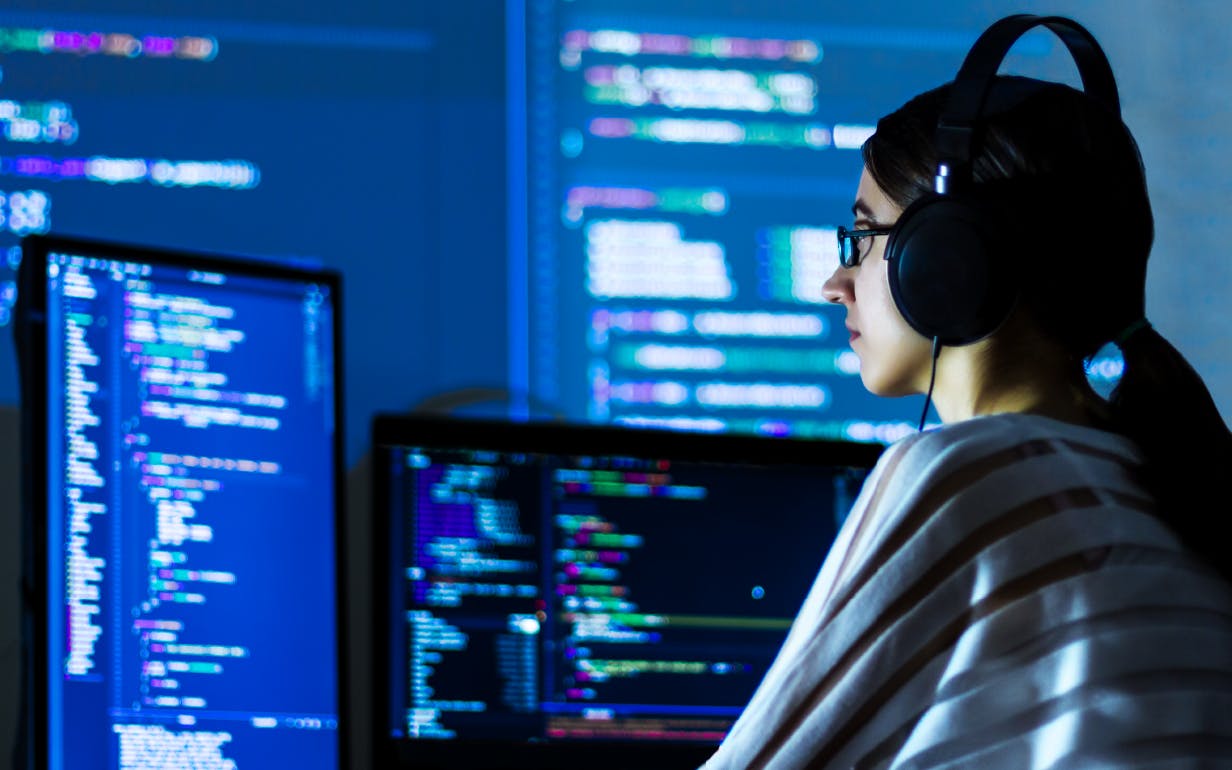
If you are working on developing an app or game, one of the most important things to do is optimize it in terms of performance. You have to think of the various aspects including memory usage, file size, time taken to load elements, performance etc., but to add more value to your app, you can equally focus on the performance aspect and make sure nothing slows down your application.
This tutorial explains how you can use Arrays in Swift along with ContiguousArray.3
What is array in Swift and how do they function?
The most common data type in an app is the array. You can organize your app's data using arrays. Arrays hold single-type elements, known as the array's element type. It is possible to store any type of element in an array, from integers to strings and to classes.
Swift makes creating arrays easier with array literals. You can simply surround a list of values with square brackets. Swift creates an array containing specified values, inferring the array's element type automatically. For example:
// An array of 'Int' elements
let evenNumbers = [2, 4, 6, 8, 10, 12, 14]
// An array of 'String' elements
let country= ["India", "America", "Japan"]
If you specify the element type of your array in the declaration, you will be able to create an empty array. For example:
// Shortened forms are preferred
var emptyFloat: [Float] = []
// The full type name is also allowed
var emptyInt: Array<int> = Array()
You can use the Array(repeating: count:) initializer if you need your array to be pre-initialized with a fixed number of values.
var numbCounts = Array(repeating: 0, count: 5)
print(numbCounts)
// Prints "[0, 0, 0, 0, 0]"
Accessing array values
You can iterate through an array's elements using it for - in loop when performing an operation on all the elements.
for street in street_names
{
print("I don't know \(street).")
}
// Prints "I don't know Broadway."
// Prints "I don't know Abbey Road."
// Prints "I don't know Orchard Road."
If you need to check quickly whether an array contains any elements, use the isEmpty property, or if you want to know how many elements are in it, use the count property.
if evenNumbers.isEmpty
{
print("Array don't contain any even numbers.")
} else {
print("Array contains \(evenNumbers.count) even numbers.")}
// Prints "Array contains 5 even numbers."
You can access the first and last elements of an array using the first and last properties. They will be nil if the array is empty.
if let firstElement = evenNumbers.first, let lastElement = evenNumbers.last
{
print(firstElement, lastElement, separator: ", ")}
// Prints "2, 16"
print(emptyFloat.first, emptyFloat.last, separator: ", ")
// Prints "nil, nil"
You can access array elements through subscripts. Arrays with no empty elements start at index zero. Any integer can be subscripted onto an array up to its count but not beyond it. If the count is less than or equal to a negative number you will encounter a runtime error. For example:
print(evenNumbers[0], evenNumbers[3], separator: ", ")
// Prints "2, 8"
print(emptyFloat[0])
// It will trigger a runtime error: Index out of range
Adding and removing elements
Consider the case where you need to keep track of the names of all the students in a class.
It is necessary to add and remove names during the registration period as students enroll and drop out.
var student_name = ["Austin", "Bill", "Jordell"]
You can add a single element to an array by using the append(_:) method. You can add multiple elements at once by using the append(contentsOf:) method.
student_name.append("Cassidy")
student_name.append(contentsOf: ["Shakia", "Harry"])
// ["Austin", "Bill", "Jordell", "Cassidy", "Shakia", "Harry"]
You can insert a single element into a collection or array literal by using the insert(_:at:) method, or multiple elements from another collection or array literal by using the insert(contentsOf:at:) method. In order to make room, elements at that index and later indices are shifted backward.
student_name.insert("Liam", at: 3)
// ["Austin", "Bill", "Jordell","Liam", "Cassidy", "Shakia", "Harry"]
The methods remove(at:), removeSubrange(_:), and removeLast() will get used to removing elements from an array.
// Austin's family is moving to another place
student_name.remove(at: 0)
// ["Bill", "Jordell","Liam", "Cassidy", "Shakia", "Harry"]
// Harry is signing up for a different school
student_name.removeLast()
// ["Bill", "Jordell", "Liam", "Cassidy", "Shakia"]
You can assign a new value to a subscript to replace an existing element.
if let i = student_name.firstIndex(of: "Bill")
{
student_name[i] = "Billy"
}
// ["Billy", "Jordell", "Liam", "Cassidy", "Shakia"]
Swift array sorting and filtering
Sorting Array
In Swift, there are two ways of sorting array. One mutates the array, and another doesn’t. In both cases, the element in the collection had to conform to the Comparable protocol.
The relational operators *, *=, >=, and > can be used to compare types that conform to the Comparable protocol. The string is one type that confirms the Comparable protocol that is part of the standard library.
There are two sorts, sort() and sort(by:), which mutate an array. A mutable array value can be sorted by calling sort() over it.
var student_name= ["Billy", "Jordell", "Liam", "Cassidy", "Shakia"] // <1>
student_name.sort()
print(student_name)
// ["Billy", "Cassidy", "Jordell", "Liam", "Shakia"]
Filtering Array
When you create an array of elements and want to remove all elements that don't match specific criteria, you're looking for the filter(isIncluded:) method of the array, which is the method for removing all elements that do not match specific criteria.
Consider the following example, if you have an array of different words, and you want to retain only those words with more than three characters:
let words = ["hello", "world", "this", "is", "an", "example", "of", "filtering"]
let filtered = words.filter { word in
return word.count >= 3
} // filtered is ["hello", "world", "this", "example", "filtering"]
Each element in the source array is filtered using the filter(isIncluded:) method. An array will be filtered if this closure returns true. Returning false will ignore the element and prevent it from being included in the array. A filter does not modify the original Array when applied to it. This method creates a new temporary array with only the desired elements by filtering (isIncluded:) and displaying output.
What is ContiguousArray in Swift?
ContiguousArrays are specialized arrays that store their elements in contiguous memory regions. Comparatively, arrays, which can have their elements stored in either a contiguous region of memory or as an NSArray instance if their element type is a class or @objc protocol, store their elements as Arrays.
You may find that using ContiguousArray to store your array is more efficient and more predictable than using Array if your array's Element type is a class or @objc protocol. In general, Array and ContiguousArray should have similar efficiency if the array's Element type is a struct or enumeration.
ContiguousArray is a very powerful tool to use when you need to create any sort of array that elements can be added/ removed right away without the need to reindex. This is a very good solution if you do not know the size of your array in advance while adding/or removing elements in your array.
The only problem with contiguous arrays is that they are always smaller than the size you first declare them as. So if you initially declared it as an Int16 array and a large number of elements inserted into the array unexpectedly, it will resize itself up to Int32 and would possibly make copies of elements that are located far from its original memory location.
You can use a literal array to create an array. When you use an array literal, the compiler uses this initializer. So use an array literal instead of an array literal to create a new array. Then enclose each value in square brackets, comma-separated.
Here is an example of an array literal containing only strings to create an array of strings:
let ingredients: ContiguousArray = ["cocoa beans", "sugar", "cocoa butter", "salt"]
Difference between Contiguous and Non-contiguous Memory Allocation
Conclusion
In this article, we have seen what is an array along with its functioning and how to create a ContiguousArray. What is the difference between contiguous and non-contiguous memory allocation? We also saw some basic examples for demonstrating array functioning.
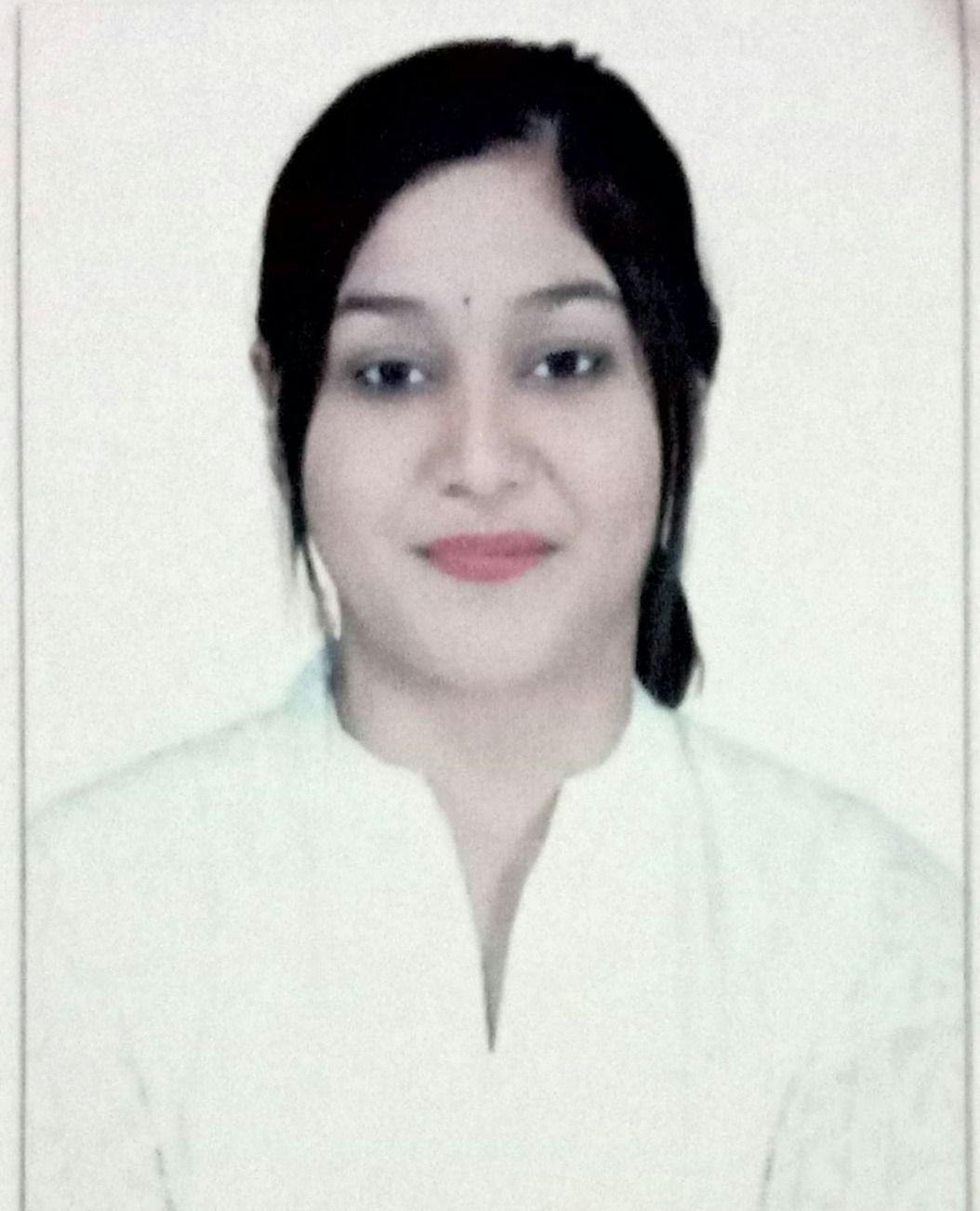
Author
Sanskriti Singh
Sanskriti is a tech writer and a freelance data scientist. She has rich experience into writing technical content and also finds interest in writing content related to mental health, productivity and self improvement.