How to Create a RESTful API Using the Flask Framework?
•8 min read
- Languages, frameworks, tools, and trends
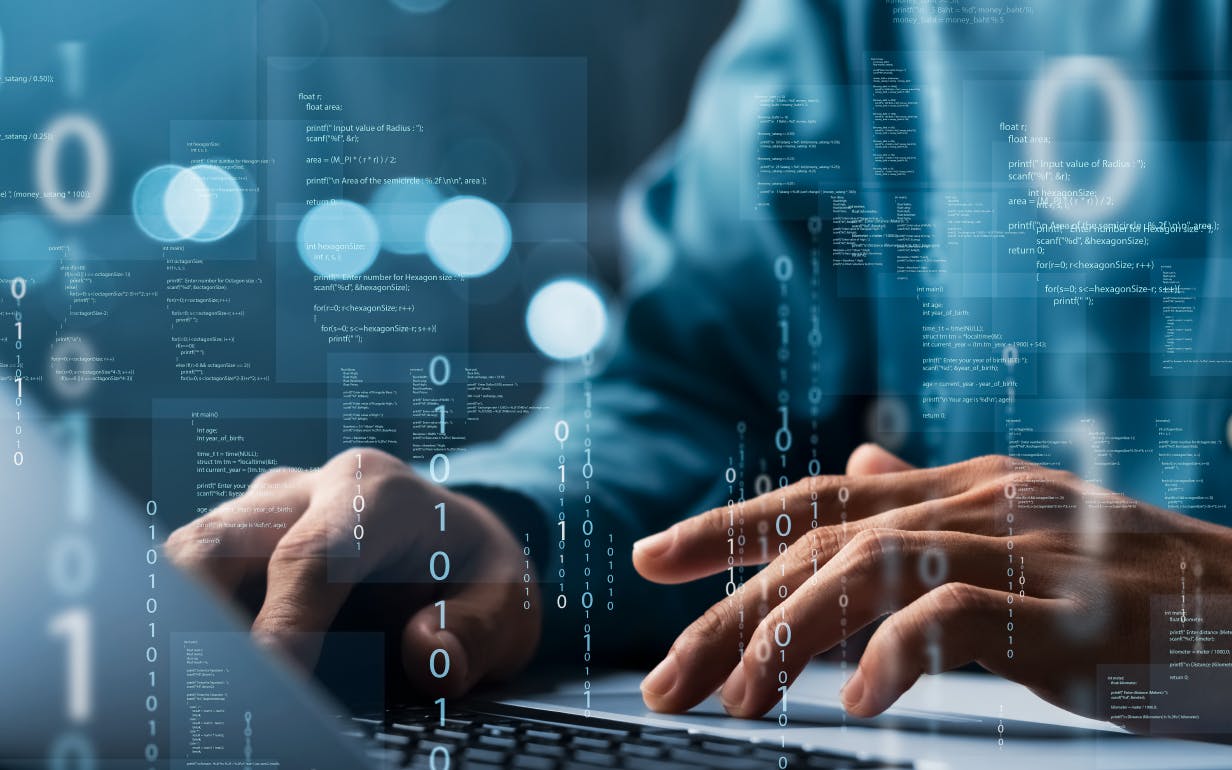
In this article, we will discuss REST API and how to create a RESTful API with the help of the Flask framework. The REST API is also known as RESTful API. Currently, REST APIs play a significant role in communication channels. It has become the standard factor to pass information across the different systems in the JSON format.
Why Python?
Recently, developers prefer to use Python for developing applications. As per the analysis of StackOverflow, Python is known to be one of the fast-growing programming languages. The language shows signs of adoption as per GitHub. There is a huge community of developers that are bagging for Python. The Python software foundation also gives great documentation where adopters can learn it fast.
What is an API?
A web API allows information or functionality to be manipulated by other programs with the help of the internet. For example, in Twitter’s web API, you can start writing a program in a language that can perform related tasks.
The term API is abbreviated for Application Programming Interface. It refers to a section of a computer program designed to be manipulated by another program, as opposed to an interface designed for human manipulation. Computer programs frequently require communication among each other or with the underlying operating system. The APIs are one way of doing the above.
When to create an API?
You should consider creating an API under the following circumstances:
- Your users require access to your data in real-time, like displaying it as a part of an application or displaying it on another website.
- Your data is frequently updated or changed.
- Your users require performing actions rather than retrieving data like updating, contributing, or deletion of data.
- Your dataset becomes larger, making download tougher.
- Your user has access to only one part of the data at a specified time.
An API can be an ideal method when you want to share the data you have. But, when the size is relatively smaller, you can give a data dump in the form of a downloadable XML, SQLite, JSON, or CSV file. As per your resources, this will help you in disabling the downloading size by a few GBs.
You can provide API and data dump for users to find one or more matches. Open Library is an example that provides a clear understanding of both API and data dump. Each of them serves different use cases for different users.
Terminologies of API:
When you are creating or using an API, you should understand these terminologies:
HTTP: Hypertext Transfer Protocol is the primary reason for communication between the data on the web. It implements many methods that define the data movement path and also defines what happens to the data. The common ones are GET - pulling data from a server, and POST - pushing the data to the server.
JSON: JavaScript Object Notation is a text-based data storage method designed for easy readability for both machines and humans. It is the most common format for returning the data through API and XML.
URL: Uniform Resource Locator is an address of resistance on the website. It contains a protocol, domain, and path. It describes the location of a specific resource like a webpage. While understanding APIs, you should look for requests, URI, or endpoints for describing adjacent ideas.
REST: Representational State Transfer describes some common practices for implementing APIs. It is designed with some principles known as REST APIs. When the API outlines some principles to the users, there usually is a lot of disagreement.
What is REST API?
REST API can be abbreviated as Representational State Transfer. It is also known as RESTful API. It means when a client machine places a request for obtaining information about resources from the server, the server machine will transfer the current resource state to the client machine.
In the above image, you can see that the client machine is your computer from where you can request data for a database server. All the communications will be done over the REST APIs and you will be provided with the required outcome.
Here are a few methods which are used along with the REST API:
GET: The GET method is used by the client machine for selecting or retrieving data from the database server.
POST: The POST method is used by the client machine for sending or writing data on the database server.
PUT: The PUT method is used by the client machine for updating existing data on the database server.
DELETE: The DELETE method is used by the client machine for deleting the existing data on the database server.
The server is currently not limited to a database server. But it can also be another machine that will read and write data from a flat file.
Why Flask?
Django and Flask are the two most popular frameworks for web development in Python. Django is the older one that is more mature and popular. The Flask is less popular but not that far behind. Though Django is older and has a higher community, Flask has its strength.
From scratch, Flask was created keeping simplicity and scalability in mind. Flask applications are meant to be lightweight. The code for it is very simple but extensive. Flask will always make decisions in favor of the developer. It also has documentation that will address where the data should start with the developer.
Creating a RESTful API using the Flask API Framework
How to create and test a REST API?
You can create APIs using any programming language. We will create an API using the Flask framework. Flask is a lightweight web application development framework that does the heavy lifting to create a server and allows us to focus on the business logic of creating the APIs.
When we want to consume these APIs, which are also known as endpoints, we need our clients to place their requests to the server for resources. The client should be a different application that will make the request. But, it is not possible for developing an entire application for testing your API. For that, you should have another tool that will test our endpoints once the development is over.
Creating REST API using Flask API Framework in Python
We can now look at how to create an API using Flask API Framework and test it using Postman. For instance, we can read and write data to a CSV file that has information about users. The file will have details about the users - their name, age, and their qualification.
Importing the modules and initializing an application
We can now write code by importing the Flash modules and initializing the web application. You can use the simple snippet for the initialization process:
from flask import
Flask
from flask_restful_api import API, Resource,
reqparse
import pandas as pd
app = Flask(_user_)
api = API(app)
Creating the RESTful API endpoints
When the initialization is over, we can write the code for the endpoints that will let us consume the APIs. We will read and write data from only one file and have only one endpoint. The URL for this endpoint can be localhost/users when you are testing with the local machine, or "www.your-website-name.com/users" when you have deployed it online.
When you want to let the Flask understand which code to execute, you can call the endpoint. Below is the snippet for the code block in the Python class and add the information which will let the Flask know which class to bind with which endpoint.
class
Users(Resources):
# Writing a method for fetching the data from the CSV file
def get(self);
pass
# Writing a method for writing data into the CSV file
def post(self);
pass
# Writing a method for updating data into the CSV file
def delete(self);
pass
# Adding URL endpoints
api.add_resource(Users, ‘/users’)
You can see how the new classes are defined with the name Users and how the methods are initialized into the class for specific tasks like getting, posting, and deleting. When the class is created along with the definition of a method, the Flask will now know which endpoint is associated with which class.
When the ‘/users’ endpoint is called, the Flask will know which class must route to while the operation is being performed. You can achieve it using the method add_resource and pass the class and the endpoint to it. Whereas when you have more than one endpoint, you can add more classes and then add resources as per your need.
Writing methods for reading and writing data
After creating the class and binding it to the endpoint, you can write methods like reading, writing, deleting, and updating from the CSV file. When you want to read data from the CSV file, we can use the Pandas library. It will make it easier to deal with the data from flat files.
We can try to understand the three methods that we have used in the above example:
- get - get is the simplest method that will read the data from the CSV file and convert it into a Python dictionary. Also, it will return it to the user. The status code 200 will show that the request is a success.
- post - the post is a method to create a new user in the CSV file. When you want to create a new user you should fill in the details as a parameter while calling the API endpoint. The parameters will then be parsed, and the creation of a new data frame will be done for storing data. The status code 201 will show that the request for creation is a success.
- delete - delete is a method for deleting records from the CSV file. Generally, while performing such operations, you should pass a unique identifier, but also can remove the name of the user from the file.
At last, when you have completed all the methods, you should run the application and start putting it on the web application. You can implement this and run the application. This will run the web application on the development server and you can see the URL endpoint console.
Testing the endpoints using the Postman
Once when the web application server is up and running, we can now start testing it. You should create a new GET request in Postman. The endpoint of the URL will return the data from the CSV file with a status of 200. Also, when you want to add a new user to the file, you can use the post method and provide the details as parameters.
We understood more about the REST API and how to create one using the Flask framework in Python. You can also understand it better with the above-mentioned example which will implement different classes, security features, and endpoints. RESTful API is an excellent way to communicate between systems and also helps in creating a foundation for a better understanding of how these APIs work.
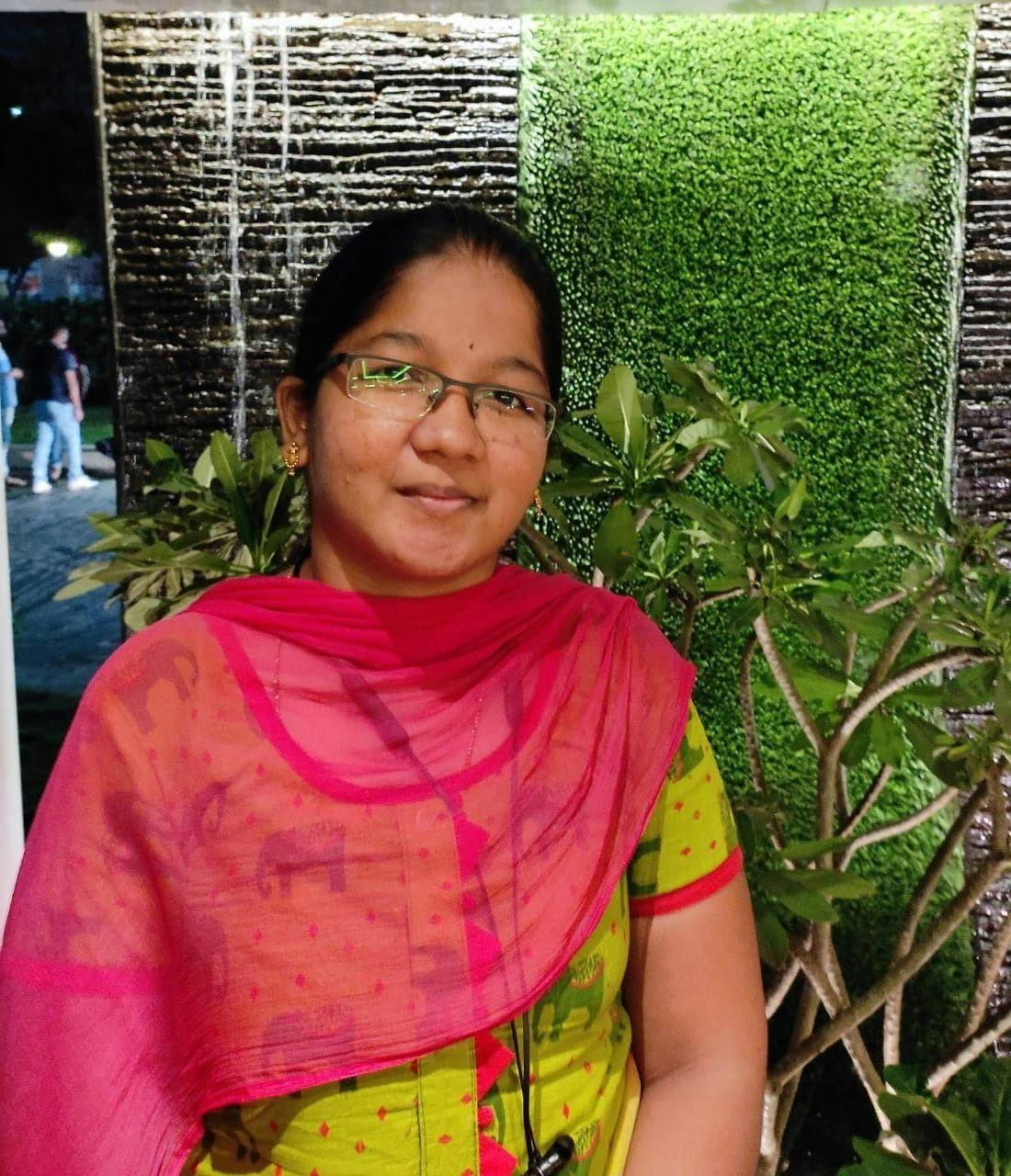
Author
Aswini R
Aswini is an experienced technical content writer. She has a reputation for creating engaging, knowledge-rich content. An avid reader, she enjoys staying abreast of the latest tech trends.