What is Apache Maven | A Build Automation Tool for Java
•7 min read
- Languages, frameworks, tools, and trends
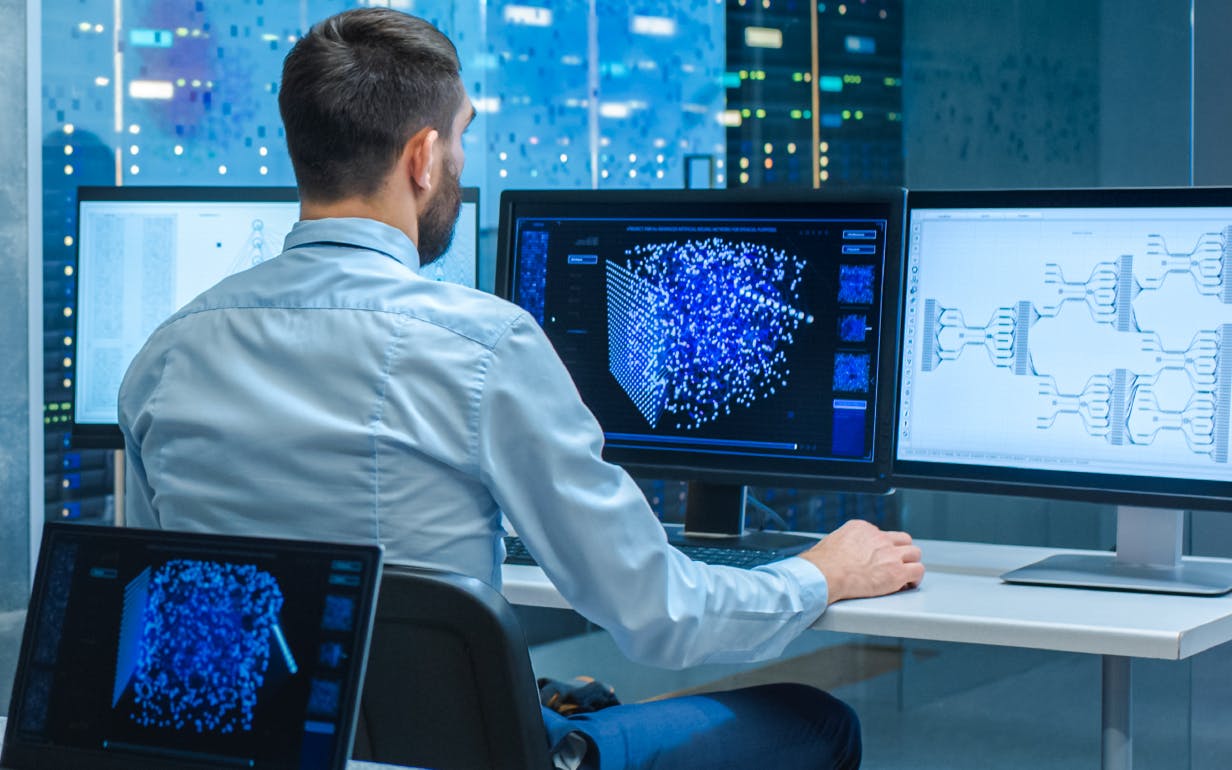
Maven is used for a wide range of projects in the modern software industry. A majority of developers around the world aim to master the language to not just find secure top opportunities but also develop, manage, and maintain a wide variety of Java-based projects in the enterprise domain. It significantly extends the development capabilities of professionals by improving the core abilities of building business applications.
Maven is controlled via the Project Object Model file (POM). We often need to deal with multiple dependencies when working with Java frameworks. Before Maven, dependencies that were nothing, but JAR (Java ARchive) files had to be manually added to our framework. You will also need to manage the software upgrade within your project. Mastering the management tool can significantly help to scale career opportunities and secure top developer jobs.
So, let’s get started!
What is Maven in Java?
Maven is a project and build management tool. It is most commonly used in Java-based frameworks. Apache Software Foundation developed it. Maven is a Yiddish word that means "gatherer of information".
Maven automatically downloads project JARs, libraries, and other files. In the pom.xml file, only information about the version of the software and the type of dependencies must be included. Maven can manage projects in Ruby, C#, and other languages. It is responsible for building projects, their dependencies, and documenting them.
ANT (Another Neat Tool), another tool created by Apache Software Foundation, is used to build and deploy projects. Maven, however, is much more potent than ANT. Maven, like ANT, has simplified the process of building. Maven, therefore, has simplified the lives of developers.
Key features of Maven
- Simple project setup
- Simplifies the process of building projects by hiding unnecessary details
- Uniform build system in which a standard strategy is used
- Dependency management, which includes automatic updating
- The ability to manage multiple projects at once
- Dynamic downloading of required Java plug-ins and libraries from the Maven repository
- Information about the quality of your project, including dependency lists, cross-referenced sources, and unit test report
What are the core concepts in Maven?
POM Files: Project Object Model Files (POM) files are XML files that contain information about the project and configuration information like dependencies, source directory and plug-ins, goals, etc. Maven uses these files to build the project. Maven needs a POM file in order to execute Maven commands. Maven uses pom.xml to perform its operations and configuration.
Build Plug-ins: Maven can be described as a plug-in execution system. These plug-ins can be used to accomplish a specific task. A plug-in can be added to the pom.xml file. Maven provides a variety of plug-ins that you can use. You can also create your own plug-ins in Java.
Build Profiles: If you want to build your project in multiple ways. You can customize your build for different environments, such as production and development. There are three types: Global, Per profile, and Per user.
Build life cycles, phases, and goals: A build lifecycle is an organized sequence of phases. It determines the order in which the goals will be accomplished. Each phase of a build phase has a set of goals. Execution of one life cycle means that all life cycle phases are completed. When a build phase has been executed, all previous build phases in the predetermined sequence of build phases are also executed.
Dependencies and repositories: Dependencies are external Java libraries that are required for the project. Repositories are directories containing packaged JAR files. Your local repository is a directory on your computer's hard drive. If dependencies are not located in the Maven local repository, Maven will download them from a central Maven repository to your local repository.
Maven pom.xml file
POM stands for Project Object Model. This pom.xml file contains information about the project, configuration information, and dependencies. This includes the build directory, source directory, test source directory, goals, plug-in, and so on.
Maven reads through the pom.xml and then executes the goal.
You will need these elements to create a simple pom.xml:
project: This is the root element of the pom.xml file.
groupId: It is the sub-element of the project. It defines the id for the project group.
modelVersion: It is the sub-element of the project. It defines the model version.
artifactId: It is the sub-element of the project. It defines the ID for the project. Artifacts can be either produced or utilized by a project. Examples of artifacts include JARs, binary and source distributions, and WARs.
version: It is the sub-element of the project. It defines the version of the artifact under a given group.
A code example of a pom.xml file is shown below:
pom.xml file contains some additional elements:
scope: It specifies the scope of the Maven project.
packaging: It specifies the packaging type such as jar, war, etc.
url: It defines the URL of the project.
name: It specifies the name of the project.
dependencies: It defines dependencies for the Maven project.
dependency: specifies a dependency. It is used within dependencies.
A code example of a pom.xml file with additional elements:
Maven Repository
A repository is a directory on your machine. The project's jars, the plug-ins, or other materials related to the project are stored here.
Maven Repository can be of three types:
- Local Repository
- Central Repository
- Remote Repository
When a dependency has to be searched, it initially searches the local repository, then the central repository, and at last the remote repository.
If the dependencies aren't discovered in one of the three repositories, the user is informed with an error message, and the process ceases.
Local Repository
Maven's local repository is a directory on your machine in which all the project components are kept. When the Maven build is finished, Maven automatically downloads all the dependencies jars to Maven's local repository.
The default maven local repository is the user_home/m2 directory.
The default path can be modified in settings.xml.
Central Repository
If dependencies are not found in the local repository, Maven then searches the central repository. Maven then downloads the dependencies to the local repository.
Apache Maven group developed the central repository, and it is hosted on the web.
Like the local repository, the path of the central repository can be modified in setting.xml.
Remote Repository
It is similar to the central repository. When Maven wants to download a dependency, it goes to a remote repository. The remote repository is present on a web server and is widely used to host the internal projects of an organization.
How can Maven help in the development process?
Maven makes it easier to create a Java-based project when you work as a Java developer. Maven configuration allows easy access to any new feature that has been created or added to the project. This increases project performance and speeds up the building process. Maven's main feature is the ability to automatically download project dependencies libraries.
Here are some examples of popular IDEs that support development with Maven Framework.
- Eclipse
- IntelliJ IDEA
- NetBeans
- JBuilder
How to install Maven?
Maven is a Java project; therefore, you'll need a JDK installed on your machine before installing it.
Once you have installed the Java development environment, you can start installing Maven in a couple of steps:
Download the most recent Maven release.
Download and save the apache.maven.zip document to a suitable location.
Add a MAVEN_HOME system variable, and then point it to the Maven folder.
Add %MAVEN_HOME%\bin To PATH
Now you will have access to mvn commands. Start a new command prompt, and type mvn –version to verify successful installation.
Advantages of Maven
- Maven manages all the processes, such as building, releasing, documentation, and distribution of the projects
- Maven automatically includes all necessary dependencies for your project after reading the pom.xml file.
- It is very easy to create projects using jar, war, and so on as per requirements.
- Maven allows you to start projects easily in various environments, and you don't have to worry about dependency injection, builds, processing, etc.
- The process of adding a new dependency can be extremely easy. You just need to write the code for the dependency in the pom.xml file.
Disadvantages of Maven
- Maven requires maven installation on the system in order to work as well as the maven plug-in that is required for the ide.
- Dependency cannot be added if the Maven code for an existing dependency is not available.
Conclusion
Maven is a management and automation tool. Maven, a well-known open-source build tool for enterprise Java projects, is specifically designed to take most of the effort out of the building process.
Maven utilizes a declarative style of approach which means that the project's structure and content are described instead of the task-based approach employed in Ant. This makes it easier to enforce corporate-wide standardization of development and decreases the amount of time required to create as well as maintain the build scripts.
When you employ Maven and create an established project object model, Maven can then apply cross-cutting logic using a set of shared plug-ins. If you have multi-team development environments, Maven can set up the process to function according to guidelines briefly.
Since most settings for projects are straightforward and reusable, Maven makes the developer's life easier when it comes to making documents, checking, and testing automation configurations.
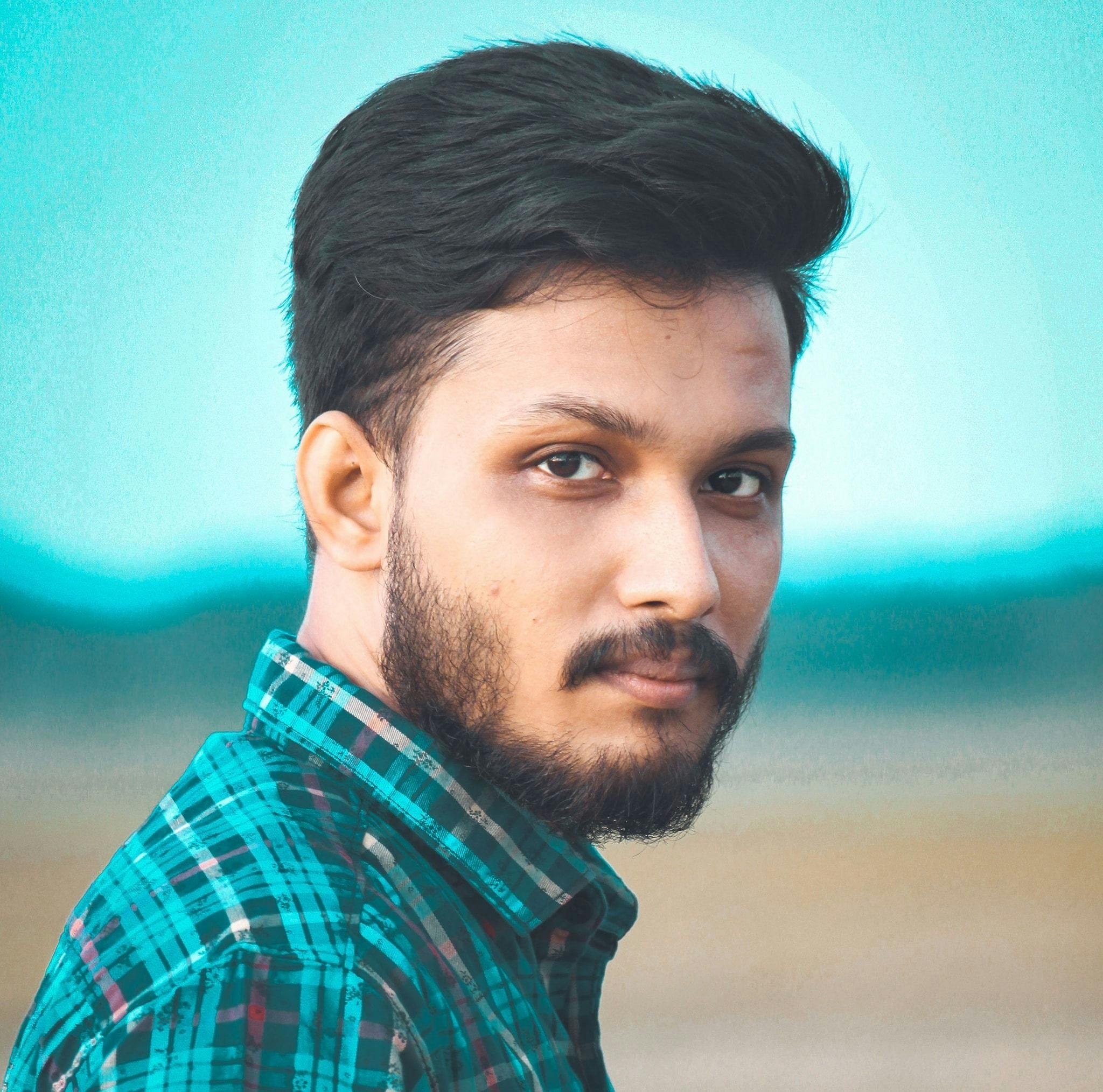
Author
Soumyakanti Ray
Soumyakanti Ray is a writer, blogger, and SEO analyst with over nine years of experience. His work featured in top publications like The Verge, India Today, and NDTV. He is a big-time foodie. He loves to play cricket, football, and video games in his free time.